JavaScript: Compute the sum of two parts and store into an array of size two
JavaScript Basic: Exercise-85 with Solution
Split Array into Two Sums Alternating Elements
Write a JavaScript program to divide a given array of positive integers into two parts. First element belongs to the first part, second element belongs to the second part, and third element belongs to the first part and so on. Now compute the sum of two parts and store it in an array of size two.
Visual Presentation:
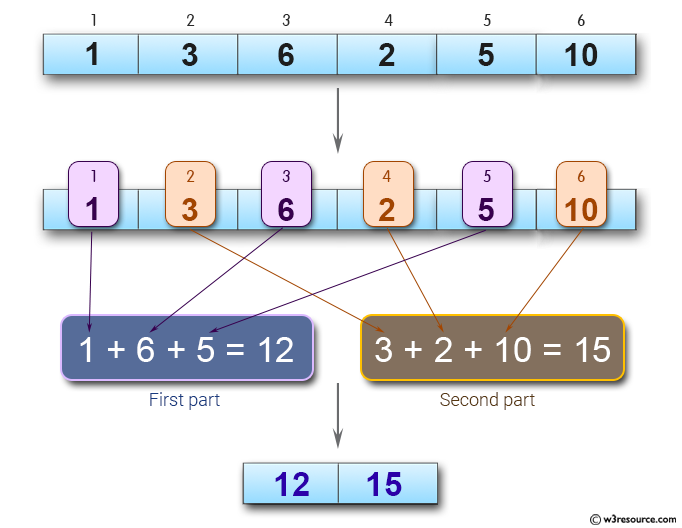
Sample Solution:
JavaScript Code:
// Function to calculate alternate sums of elements in an array
function alternate_Sums(arr) {
var result = [0, 0]; // Initialize an array to store alternate sums
for(var i = 0; i < arr.length; i++)
{
if(i % 2) result[1] += arr[i]; // If index is odd, add the element to the second sum
else
result[0] += arr[i]; // If index is even, add the element to the first sum
}
return result; // Return the array of alternate sums
}
// Example usage
console.log(alternate_Sums([1, 3, 6, 2, 5, 10])); // Output: [14, 8]
Output:
[12,15]
Live Demo:
See the Pen javascript-basic-exercise-85 by w3resource (@w3resource) on CodePen.
Flowchart:
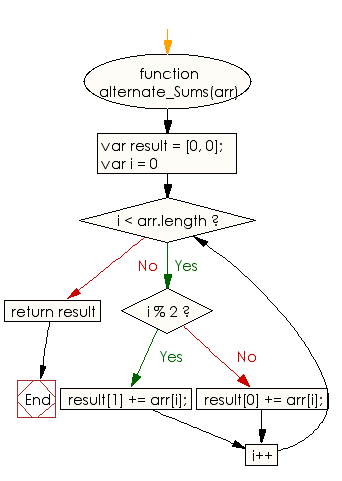
ES6 Version:
// ES6 version with comments
// Function to calculate alternate sums of elements in an array
const alternate_Sums = (arr) => {
var result = [0, 0]; // Initialize an array to store the alternate sums [sum1, sum2]
for(var i = 0; i < arr.length; i++)
{
if(i % 2) result[1] += arr[i]; // If the index is odd, add the element to the second sum
else
result[0] += arr[i]; // If the index is even, add the element to the first sum
}
return result; // Return the array with alternate sums
};
// Example usage
console.log(alternate_Sums([1, 3, 6, 2, 5, 10]));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript to replace each character of a given string by the next one in the English alphabet.
Next: JavaScript program to find the types of a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-basic-exercise-85.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics