JavaScript: Find the types of a specified angle
JavaScript Basic: Exercise-86 with Solution
Find Type of Given Angle
Write a JavaScript program to find the types of a given angle.
Types of angles:
• Acute angle: An angle between 0 and 90 degrees.
• Right angle: An 90 degree angle.
• Obtuse angle: An angle between 90 and 180 degrees.
• Straight angle: A 180 degree angle.
Visual Presentation:
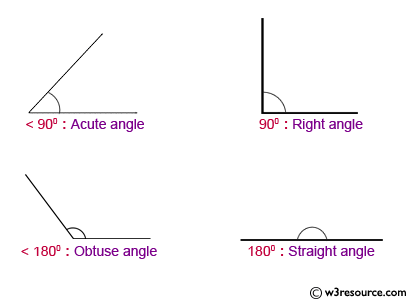
Sample Solution:
JavaScript Code:
// Function to determine the type of angle based on its measure
function angle_Type(angle) {
if(angle < 90) {
return "Acute angle."; // Return this message if the angle is less than 90 degrees
}
if(angle === 90) {
return "Right angle."; // Return this message if the angle is exactly 90 degrees
}
if(angle < 180) {
return "Obtuse angle."; // Return this message if the angle is less than 180 degrees but not 90 degrees
}
return "Straight angle."; // Return this message if the angle is exactly 180 degrees
}
// Example usage
console.log(angle_Type(47)); // Acute angle.
console.log(angle_Type(90)); // Right angle.
console.log(angle_Type(145)); // Obtuse angle.
console.log(angle_Type(180)); // Straight angle.
Output:
Acute angle. Right angle. Obtuse angle. Straight angle.
Live Demo:
See the Pen javascript-basic-exercise-85 by w3resource (@w3resource) on CodePen.
Flowchart:
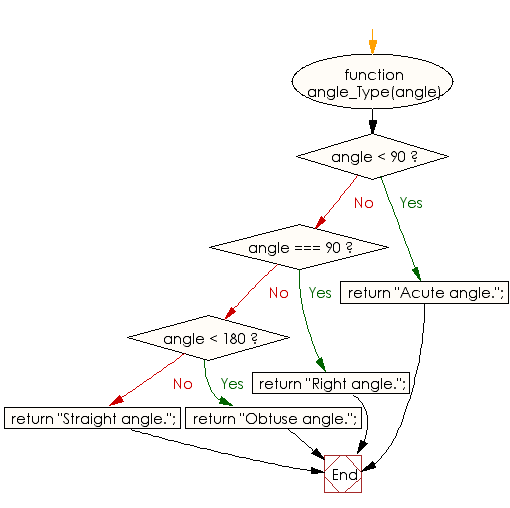
ES6 Version:
// Function to determine the type of angle based on its measure
const angle_Type = (angle) => {
if (angle < 90) {
return "Acute angle."; // Return this message if the angle is less than 90 degrees
}
if (angle === 90) {
return "Right angle."; // Return this message if the angle is exactly 90 degrees
}
if (angle < 180) {
return "Obtuse angle."; // Return this message if the angle is less than 180 degrees but not 90 degrees
}
return "Straight angle."; // Return this message if the angle is exactly 180 degrees
};
// Example usage
console.log(angle_Type(47)); // Acute angle.
console.log(angle_Type(90)); // Right angle.
console.log(angle_Type(145)); // Obtuse angle.
console.log(angle_Type(180)); // Straight angle.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that accepts an angle in degrees and categorizes it as acute, right, obtuse, or straight, ensuring valid input.
- Write a JavaScript function that determines the type of an angle based on its measure and returns a descriptive string.
- Write a JavaScript program that validates an angle’s value (0–180) and outputs its classification using conditional statements.
Go to:
PREV : Split Array into Two Sums Alternating Elements.
NEXT : Check if Two Arrays Are Similar with One Swap.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.