JavaScript: Get the current date
JavaScript Datetime: Exercise-2 with Solution
Get Current Date
Write a JavaScript function to get the current date.
Test Data :
console.log(curday('/'));
console.log(curday('-'));
Output :
"11/13/2014"
"11-13-2014"
Sample Solution:
JavaScript Code:
// Define a JavaScript function called curday with a parameter sp
var curday = function(sp){
// Create a new Date object representing today's date
today = new Date();
// Get the day of the month
var dd = today.getDate();
// Get the month, adding 1 because JavaScript months are zero-based (January is 0)
var mm = today.getMonth()+1; // As January is 0.
// Get the full year
var yyyy = today.getFullYear();
// If the day of the month is less than 10, prepend '0' to it to ensure two digits
if(dd<10) dd='0'+dd;
// If the month is less than 10, prepend '0' to it to ensure two digits
if(mm<10) mm='0'+mm;
// Return the formatted date string with the specified separator
return (mm+sp+dd+sp+yyyy);
};
// Output today's date formatted with '/' separator
console.log(curday('/'));
// Output today's date formatted with '-' separator
console.log(curday('-'));
Output:
06/19/2018 06-19-2018
Explanation:
In the exercise above -
- The code defines a JavaScript function named "curday()" with a single parameter 'sp', which represents the separator to be used in the formatted date string.
- Inside the function:
- It creates a new Date object called "today", representing the current date and time.
- It extracts the day of the month (dd), month (mm), and full year (yyyy) from the "today" object.
- Since JavaScript months are zero-based (January is 0), it adds 1 to the month value to get the correct month.
- It checks if the day of the month or the month itself is less than 10. If so, it prepends '0' to ensure that they are represented with two digits.
- After formatting the date components, the function returns a string representing the current date in the format 'mm/sp/dd/sp/yyyy', where 'sp' is the separator provided as an argument.
- The code then calls the "curday()" function twice:
- First with '/' separator and logs the result to the console using console.log(curday('/'));.
- Second with '-' separator and logs the result to the console using console.log(curday('-'));.
Flowchart:
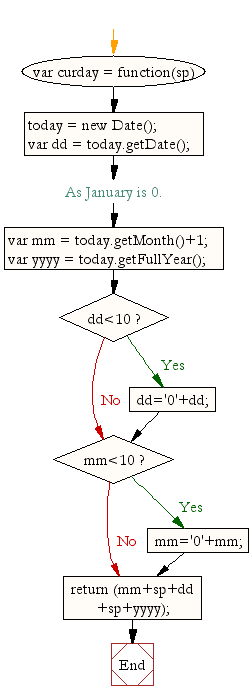
Live Demo:
See the Pen JavaScript - Get the current date-date-ex- 2 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the current date formatted with a given separator between month, day, and year.
- Write a JavaScript function that outputs the current date in both local and UTC formats.
- Write a JavaScript function that retrieves the current date and formats it in MM-DD-YYYY style using string manipulation.
- Write a JavaScript function that constructs a Date object for the current date and returns it as an ISO formatted string with custom separators.
Go to:
PREV : Check Date Object.
NEXT : Days in Month.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.