JavaScript: Get uppercase Ante meridiem and Post meridiem
JavaScript Datetime: Exercise-31 with Solution
Uppercase AM/PM
Write a JavaScript function to get uppercase Ante meridiem and Post meridiem.
Sample Solution:
JavaScript Code:
// Define a JavaScript function called upper_am_pm with parameter dt (date)
function upper_am_pm(dt)
{
// Check if the hour value of the provided date is less than 12
// If true, return 'AM'; otherwise, return 'PM'
return dt.getHours() < 12 ? 'AM' : 'PM';
}
// Create a new Date object representing the current date
dt = new Date();
// Output 'AM' or 'PM' based on whether the hour value of the current date is less than 12
console.log(upper_am_pm(dt));
// Create a new Date object representing November 1, 1989
dt = new Date(1989, 10, 1);
// Output 'AM' or 'PM' based on whether the hour value of November 1, 1989, is less than 12
console.log(upper_am_pm(dt));
Output:
PM AM
Explanation:
In the exercise above,
- The code defines a JavaScript function named "upper_am_pm()" with one parameter 'dt', representing a Date object.
- Inside the upper_am_pm function:
- It checks if the hour value of the provided Date object "dt" (obtained using "getHours()" method) is less than 12.
- If the hour value is less than 12, it returns 'AM', indicating the time is in the morning.
- Otherwise, it returns 'PM', indicating the time is in the afternoon or evening.
- The code then demonstrates the usage of the "upper_am_pm()" function:
- It creates a new Date object 'dt' representing the current date using 'new Date()'.
- It outputs 'AM' or 'PM' based on whether the hour value of the current date is less than 12 by calling the "upper_am_pm()" function with 'dt' and logging the result to the console.
- It creates another new Date object "dt" representing November 1, 1989, using 'new Date(1989, 10, 1)'.
- It outputs 'AM' or 'PM' based on whether the hour value of November 1, 1989, is less than 12 by calling the "upper_am_pm()" function with 'dt' and logging the result to the console.
Flowchart:
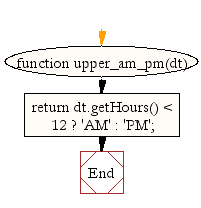
Live Demo:
See the Pen JavaScript - Get uppercase Ante meridiem and Post meridiem-date-ex-31 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns "AM" or "PM" in uppercase based on the provided Date object's hour.
- Write a JavaScript function that converts a 12-hour time string with lowercase am/pm to uppercase.
- Write a JavaScript function that validates the Date object and outputs the uppercase meridiem correctly.
- Write a JavaScript function that uses conditional operators to determine and return "AM" or "PM" in uppercase.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get lowercase Ante meridiem and Post meridiem.
Next: Write a JavaScript function to swatch Internet time (000 through 999).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.