JavaScript: swatch Internet time
JavaScript Datetime: Exercise-32 with Solution
Write a JavaScript function to swatch Internet time (000 through 999).
Test Data :
dt = new Date(1989, 10, 1);
console.log(internet_time(dt));
812
Sample Solution:
JavaScript Code:
// Define a JavaScript function called internet_time with parameter dt (date)
function internet_time(dt)
{
// Calculate the Internet time (Swatch Internet Time) based on the provided date
// The formula involves dividing the UTC hours, minutes, and seconds by their respective factors and then multiplying by 1000/24 to get the time in beats
return Math.floor((((dt.getUTCHours() + 1) % 24) + dt.getUTCMinutes() / 60 + dt.getUTCSeconds() / 3600) * 1000 / 24);
}
// Create a new Date object representing the current date
dt = new Date();
// Output the Internet time for the current date
console.log(internet_time(dt));
// Create a new Date object representing November 1, 1989
dt = new Date(1989, 10, 1);
// Output the Internet time for November 1, 1989
console.log(internet_time(dt));
Output:
430 812
Explanation:
In the exercise above,
- The code defines a JavaScript function named "internet_time()" with one parameter 'dt', representing a Date object.
- Inside the internet_time function:
- It calculates the Internet time (also known as Swatch Internet Time) based on the provided UTC time.
- The calculation involves:
- Adding 1 to the UTC hours, taking the modulus of 24 (to ensure it stays within the range of 0-23).
- Adding the UTC minutes divided by 60 (converted to decimal) and the UTC seconds divided by 3600 (converted to decimal).
- Multiplying the result by 1000/24 to convert it to beats (where 1000 beats correspond to 24 hours).
- The code then demonstrates the usage of the "internet_time()" function:
- It creates a new Date object "dt" representing the current date using 'new Date()'.
- It calculates and outputs the Internet time for the current date by calling the "internet_time()" function with 'dt' and logging the result to the console.
- It creates another new Date object 'dt' representing November 1, 1989, using 'new Date(1989, 10, 1)'.
- It calculates and outputs the Internet time for November 1, 1989, by calling the "internet_time()" function with 'dt' and logging the result to the console.
Flowchart:
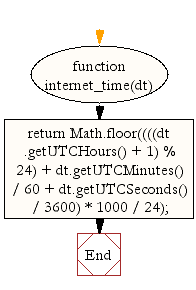
Live Demo:
See the Pen JavaScript - swatch Internet time-date-ex-32 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get uppercase Ante meridiem and Post meridiem.
Next: Write a JavaScript function to get 12-hour format of an hour with leading zeros.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-date-exercise-32.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics