JavaScript: Get the month name from a particular date
JavaScript Datetime: Exercise-4 with Solution
Month Name from Date
Write a JavaScript function to get the month name from a particular date.
Test Data:
console.log(month_name(new Date("10/11/2009")));
console.log(month_name(new Date("11/13/2014")));
Output :
"October"
"November"
Sample Solution:
JavaScript Code:
// Define a JavaScript function called month_name with parameter dt
var month_name = function(dt){
// Define an array containing names of months
mlist = [ "January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December" ];
// Return the name of the month corresponding to the month index of the provided date
return mlist[dt.getMonth()];
};
// Output the name of the month for the provided date "10/11/2009"
console.log(month_name(new Date("10/11/2009")));
// Output the name of the month for the provided date "11/13/2014"
console.log(month_name(new Date("11/13/2014")));
Output:
October November
Explanation:
In the exercise above -
- The code defines a JavaScript function named "month_name()" with a parameter 'dt', representing a date object.
- Inside the function:
- It initializes an array 'mlist' containing the names of months from January to December.
- The function returns the name of the month corresponding to the month index of the provided date. It uses the "getMonth()" method of the 'Date' object, which returns the month as a zero-based index (0 for January, 1 for February, and so on).
- Console.log() outputs the month name for the provided date to the console.
Flowchart:
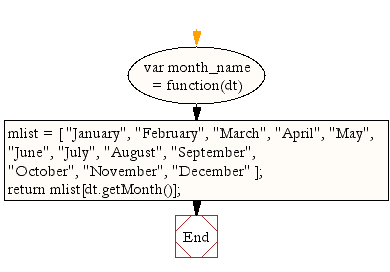
Live Demo:
See the Pen JavaScript - Get the month name from a particular date-date-ex- 4 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get the number of days in a month.
Next: Write a JavaScript function to compare dates (i.e. greater than, less than or equal to).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-date-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics