JavaScript: Display a random image by clicking on a button
JavaScript DOM: Exercise-11 with Solution
Random Image Display
Write a JavaScript program to display a random image (clicking on a button) from the following list.
Sample Image information :
"http://farm4.staticflickr.com/3691/11268502654_f28f05966c_m.jpg", width: "240", height: "160" "http://farm1.staticflickr.com/33/45336904_1aef569b30_n.jpg", width: "320", height: "195" "http://farm6.staticflickr.com/5211/5384592886_80a512e2c9.jpg", width: "500", height: "343"
Sample Solution:
HTML Code:
<!-- Declaration of HTML document type -->
<!DOCTYPE html>
<!-- Start of HTML document -->
<html>
<head>
<!-- Declaring character encoding -->
<meta charset=utf-8 />
<!-- Setting title of the document -->
<title>Display a random image.</title>
<!-- Internal CSS styling -->
<style type="text/css">
/* Styling body to have margin at the top */
body {margin-top: 30px;}
</style>
</head>
<!-- Start of body section -->
<body>
<!-- Division containing a button triggering the display_random_image function on click -->
<div>
<button id="jsstyle" onclick="display_random_image();">Show Image</button>
</div>
<!-- End of body section -->
</body>
<!-- End of HTML document -->
</html>
JavaScript Code:
// Function declaration for display_random_image
function display_random_image()
{
// Array containing image objects with src, width, and height properties
var theImages = [{
src: "http://farm4.staticflickr.com/3691/11268502654_f28f05966c_m.jpg",
width: "240",
height: "160"
}, {
src: "http://farm1.staticflickr.com/33/45336904_1aef569b30_n.jpg",
width: "320",
height: "195"
}, {
src: "http://farm6.staticflickr.com/5211/5384592886_80a512e2c9.jpg",
width: "500",
height: "343"
}];
// Array to hold pre-buffered images
var preBuffer = [];
// Loop to preload images
for (var i = 0, j = theImages.length; i < j; i++) {
preBuffer[i] = new Image();
preBuffer[i].src = theImages[i].src;
preBuffer[i].width = theImages[i].width;
preBuffer[i].height = theImages[i].height;
}
// Function to generate random integer between min and max values
function getRandomInt(min,max)
{
// Generating random integer between min and max
imn = Math.floor(Math.random() * (max - min + 1)) + min;
// Returning the pre-buffered image corresponding to the random index
return preBuffer[imn];
}
// Getting a random image from preBuffer array
var newImage = getRandomInt(0, preBuffer.length - 1);
// Removing any previous images
var images = document.getElementsByTagName('img');
var l = images.length;
for (var p = 0; p < l; p++) {
images[0].parentNode.removeChild(images[0]);
}
// Displaying the new image
document.body.appendChild(newImage);
}
Output:
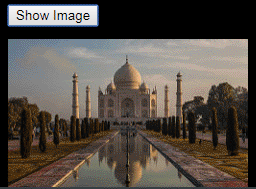
Flowchart:
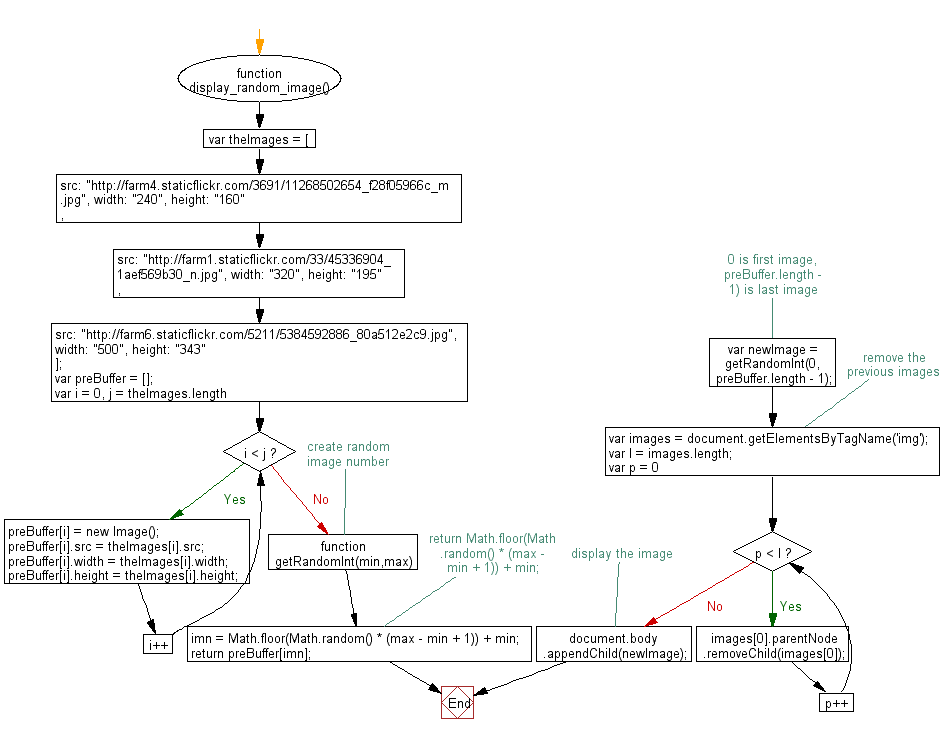
Live Demo:
See the Pen javascript-dom-exercise-11 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that displays a random image from an array of image URLs along with its specified width and height.
- Write a JavaScript function that changes the displayed image every time a button is clicked, ensuring no repetition until all images have been shown.
- Write a JavaScript function that preloads images before displaying them to prevent flickering.
- Write a JavaScript function that randomly selects an image from a list and displays it inside a specified container element.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to calculate the volume of a sphere.
Next: Write a JavaScript program to highlight the bold words of the following paragraph, on mouse over a certain link.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.