JavaScript: Highlight the bold words of a paragraph, on mouse over a certain link
JavaScript DOM: Exercise-12 with Solution
Highlight Bold on Hover
Write a JavaScript program to highlight the bold words of the following paragraph, on mouse over a certain link.
Sample link and text:
[On mouse over here bold words of the following paragraph will be highlighted]Sample Solution:
HTML Code:
<!-- Declaration of HTML document type -->
<!doctype html>
<!-- Start of HTML document -->
<html>
<!-- Start of head section -->
<head>
<!-- Declaring character encoding -->
<meta charset="UTF-8">
<!-- Setting title of the document -->
<title>Get And Style All Tags</title>
<!-- End of head section -->
</head>
<!-- Start of body section -->
<body>
<!-- Paragraph containing a link -->
<p>[<a href="#" onMouseOver="highlight()" onMouseOut="return_normal()">On mouse over here bold words of the following paragraph will be highlighted</a>]</p>
<!-- Paragraph with strong tags -->
<p><strong>We</strong> have just started <strong>this</strong> section for the users (<strong>beginner</strong> to intermediate) who <strong>want</strong> to work with <strong>various</strong> JavaScript <strong>problems</strong> and write scripts online to <strong>test</strong> their JavaScript <strong>skill</strong>.</p>
<!-- End of body section -->
</body>
<!-- End of HTML document -->
</html>
JavaScript Code:
// First create a list of all bold items
var bold_Items;
// Execute getBold_items function when window is fully loaded
window.onload = getBold_items();
// Collect all <strong> tags
function getBold_items()
{
// Get all elements with tag name 'strong' and assign them to bold_Items variable
bold_Items = document.getElementsByTagName('strong');
}
// Iterate all bold tags and change color
function highlight()
{
// Loop through each element in bold_Items
for (var i=0; i<bold_Items.length; i++)
{
// Change the color of the bold item to green
bold_Items[i].style.color = "green";
}
}
// On mouse out highlighted words become black
function return_normal()
{
// Loop through each element in bold_Items
for (var i=0; i<bold_Items.length; i++)
{
// Change the color of the bold item back to black
bold_Items[i].style.color = "black";
}
}
Output:

Flowchart:
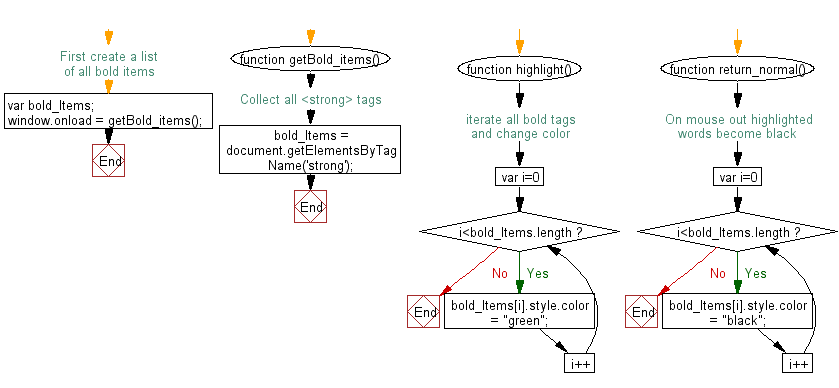
Live Demo:
See the Pen javascript-dom-exercise-12 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that highlights all bold (<strong> or <b>) elements in a paragraph when the user hovers over a specific link.
- Write a JavaScript function that toggles a highlight class on bold words when the mouse enters and leaves a parent container.
- Write a JavaScript function that changes the background color of bold text elements on hover.
- Write a JavaScript function that uses event delegation to apply a CSS style to all bold text within a section when a hover event is triggered on a button.
Go to:
PREV : Random Image Display.
NEXT : Window Resize Dimensions.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.