JavaScript: Count total number of 1s from 1 to N
JavaScript Math: Exercise-102 with Solution
Count Digit 1 in Range
Write a JavaScript program to count the number of times the digit 1 appears in 1 to a given number.
Example : 11, 1 appears 4 time between 1 to 11 [1->1, 10->1 ,11-.2]
Test Data:
(11) -> 4
(305) -> 161
(0) -> false
Sample Solution:
Solution-1
JavaScript Code:
/**
* Function to count the total number of occurrences of digit 1 from 1 to n.
* @param {number} num - The upper limit.
* @returns {number} - The total number of occurrences of digit 1.
*/
function test(num) {
if (num < 1) // If the input is less than 1, return false
return false;
var ctr = 0; // Initialize the counter for occurrences of digit 1
for (var i = 1; i <= num; i *= 10) { // Loop through each digit place
var d = i * 10; // Calculate the next digit place
// Count the occurrences of digit 1 in the current digit place
ctr += parseInt(num / d) * i + Math.min(Math.max(num % d - i + 1, 0), i);
}
return ctr; // Return the total count
}
n = 11;
console.log("n = " + n);
console.log("Total number of 1s from 1 to n: " + test(n));
n = 305;
console.log("n = " + n);
console.log("Total number of 1s from 1 to n: " + test(n));
n = 0;
console.log("n = " + n);
console.log("Total number of 1s from 1 to n: " + test(n));
Output:
n = 11 Total number of 1s from 1 to n: 4 n = 305 Total number of 1s from 1 to n: 161 n = 0 Total number of 1s from 1 to n: false
Flowchart:
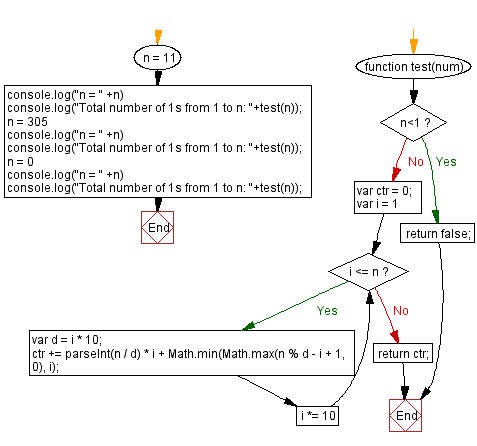
Live Demo:
See the Pen javascript-math-exercise-102 by w3resource (@w3resource) on CodePen.
Solution-2
JavaScript Code:
// Define a function named test that counts the occurrences of the digit 1 from 1 to n.
function test(n) {
// If n is less than 1, return false.
if (n < 1)
return false;
// Initialize a counter variable.
var ctr = 0;
// Iterate through powers of 10 until i exceeds n.
for (var i = 1; i <= n; i *= 10) {
// Calculate the range of digits for the current power of 10.
var d = i * 10;
// Update the counter with the number of occurrences of digit 1 in the current range.
ctr += parseInt(n / d) * i +
Math.min(Math.max(n % d - i + 1, 0), i);
}
// Return the total count of occurrences of digit 1.
return ctr;
}
// Test the function with different values of n.
n = 11;
console.log("n = " + n);
console.log("Total number of 1s from 1 to n: " + test(n));
n = 305;
console.log("n = " + n);
console.log("Total number of 1s from 1 to n: " + test(n));
n = 0;
console.log("n = " + n);
console.log("Total number of 1s from 1 to n: " + test(n));
Output:
n = 11 Total number of 1s from 1 to n: 4 n = 305 Total number of 1s from 1 to n: 161 n = 0 Total number of 1s from 1 to n: false
Flowchart:
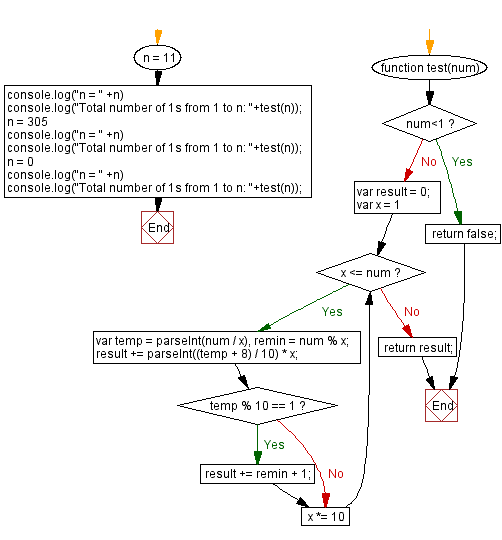
Live Demo:
See the Pen javascript-math-exercise-102-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that counts the number of times the digit '1' appears in all numbers from 1 to a given number using string conversion.
- Write a JavaScript function that iterates over each number in the range and counts the occurrences of '1' in its decimal representation.
- Write a JavaScript function that optimizes the count by analyzing each digit position across the range.
- Write a JavaScript function that validates the input and returns an error for non-positive numbers before counting.
Go to:
PREV : Find nth Ugly Number.
NEXT : String Multiplication.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.