JavaScript: Sum of all odds in a matrix
JavaScript Math: Exercise-91 with Solution
Sum of Odd Elements in Matrix
Write a JavaScript program to calculate the sum of all odd elements in a square matrix.
Test Data:
([ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]) -> 25
( [ [-1, -2], [-4, -5] ]) -> -6
Sample Solution:
JavaScript Code:
/**
* Function to calculate the sum of all odd numbers in a matrix.
* @param {number[][]} nums - The matrix containing numbers.
* @returns {number} - The sum of all odd numbers in the matrix.
*/
function test(nums) {
// Flatten the matrix into a single array
return nums.reduce((b,a) => [...b,...a], [])
// Calculate the sum of all odd numbers in the flattened array
.reduce((b,a) => !(a%2) ? b: a+b , 0);
}
// Test cases
// Test the sum of all odd numbers in the first matrix
nums = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
console.log("Sum of all odds of the said matrix: "+test(nums));
// Test the sum of all odd numbers in the second matrix
nums = [
[-1, -2],
[-4, -5]
];
console.log("Sum of all odds of the said matrix: "+test(nums));
Output:
Sum of all odds of the said matrix: 25 Sum of all odds of the said matrix: -6
Flowchart:
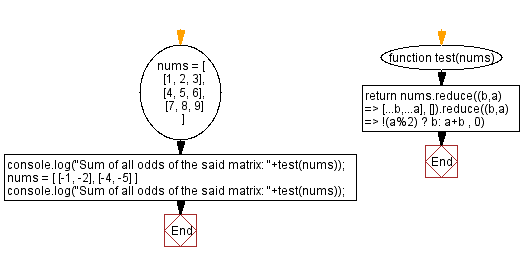
Live Demo:
See the Pen javascript-math-exercise-91 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that iterates through a square matrix and sums all the odd numbers found.
- Write a JavaScript function that uses nested loops to accumulate the sum of odd elements in a two-dimensional array.
- Write a JavaScript function that applies the filter method to each row to extract odd numbers before summing them.
- Write a JavaScript function that handles matrices with both positive and negative odd numbers while calculating the sum.
Improve this sample solution and post your code through Disqus.
Previous: Test if a number is a Harshad Number or not.
Next: Iterated Cube Root.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.