JavaScript: Iterated Cube Root
JavaScript Math: Exercise-92 with Solution
Write a Python program that takes a positive integer and calculates the cube root of the number until it is less than three. Return the number of steps to complete this process.
Test Data:
(27) -> 2
(10000) -> 2
(-100) -> "Not a positive number!"
Sample Solution:
JavaScript Code:
/**
* Function to calculate the number of iterations required to find the cube root of a number greater than or equal to 3.
* @param {number} n - The number for which cube root iterations are to be performed.
* @returns {number|string} - The number of iterations required to find the cube root, or a string indicating that the input is not a positive number.
*/
function test(n) {
let ctr = 0;
while (n >= 3) {
n = n ** (1./3.);
ctr = ctr + 1;
}
if (n < 0)
return 'Not a positive number!';
else
return ctr;
}
// Test cases
console.log("Iterated Cube Root:");
let n = 27;
console.log("n = "+n);
console.log("Number of steps to complete the said process: "+test(n));
n = 10000;
console.log("n = "+n);
console.log("Number of steps to complete the said process: "+test(n));
n = -100;
console.log("n = "+n);
console.log("Number of steps to complete the said process: "+test(n));
Output:
Iterated Cube Root: n = 27 Number of steps to complete the said process: 2 n = 10000 Number of steps to complete the said process: 2 n = -100 Number of steps to complete the said process: Not a positive number!
Flowchart:
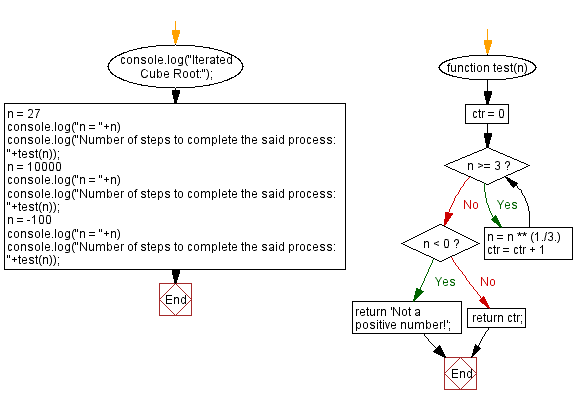
Live Demo:
See the Pen javascript-math-exercise-92 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Sum of all odds in a matrix.
Next: Check downward trend, array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-math-exercise-92.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics