JavaScript: Parameterize a string
JavaScript String: Exercise-7 with Solution
Write a JavaScript function to parameterize a string.
Test Data:
console.log(string_parameterize("Robin Singh from USA."));
"robin-singh-from-usa"
Sample Solution:
JavaScript Code:
// Define a function named string_parameterize that takes a string str1 as input
string_parameterize = function (str1) {
// Trim leading and trailing whitespace, convert to lowercase, and replace non-alphanumeric characters with an empty string
return str1.trim().toLowerCase().replace(/[^a-zA-Z0-9 -]/, "").replace(/\s/g, "-");
};
// Output the result of calling the string_parameterize function with the input "Robin Singh from USA."
console.log(string_parameterize("Robin Singh from USA."));
Output:
robin-singh-from-usa
Explanation:
In the exercise above,
- Define a function named "string_parameterize()" that takes a string 'str1' as input.
- The function first trims leading and trailing whitespace from the input string.
- It then converts the string to lowercase using the "toLowerCase()" method.
- Next, it uses a regular expression (/[^a-zA-Z0-9 -]/) to remove any characters that are not alphanumeric, spaces, or hyphens from the string, replacing them with an empty string.
- Finally, it replaces any remaining whitespace characters with hyphens using the "replace()" method.
Flowchart:
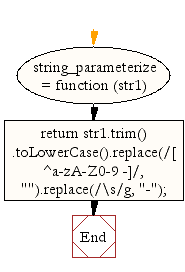
Live Demo:
See the Pen JavaScript Parameterize a string - string-ex-7 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to hide email addresses to protect from unauthorized user.
Next: Write a JavaScript function to capitalize the first letter of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-string-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics