JavaScript: Make capitalize the first letter of a string
JavaScript String: Exercise-8 with Solution
Capitalize First Letter
Write a JavaScript function to capitalize the first letter of a string.
Test Data :
console.log(capitalize('js string exercises'));
"Js string exercises"
Visual Presentation:
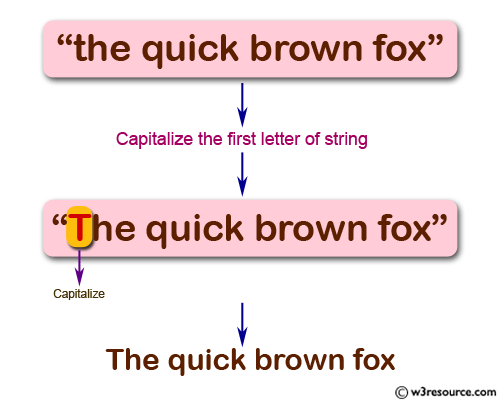
Sample Solution:
JavaScript Code:
// Define a function named capitalize that takes a string str1 as input
capitalize = function(str1){
// Capitalize the first character of the input string and concatenate it with the rest of the string starting from the second character
return str1.charAt(0).toUpperCase() + str1.slice(1);
}
// Output the result of capitalizing the string 'js string exercises'
console.log(capitalize('js string exercises'));
Output:
Js string exercises
Explanation:
In the exercise above,
- capitalize = function(str1){: This part defines a function named "capitalize()" that takes a string 'str1' as input.
- return str1.charAt(0).toUpperCase() + str1.slice(1);: Within the function, it capitalizes the first character of the input string ('str1') using the "toUpperCase()" method and concatenates it with the rest of the string starting from the second character (str1.slice(1)).
- console.log(capitalize('js string exercises'));: This line invokes the "capitalize ()" function with the argument 'js string exercises' and logs the result, which would be 'Js string exercises'.
Flowchart:
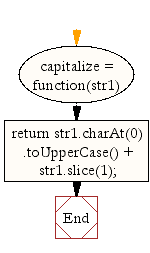
Live Demo:
See the Pen JavaScript Make capitalize the first letter of a string - string-ex-8 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that capitalizes only the first character of a given string and leaves the rest unchanged.
- Write a JavaScript function that checks if the string is empty before capitalizing the first letter.
- Write a JavaScript function that uses slice() to combine the capitalized first letter with the remainder of the string.
- Write a JavaScript function that converts the first character to uppercase using toUpperCase() and concatenates it with the substring.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to parameterize a string.
Next: Write a JavaScript function to capitalize the first letter of each word in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.