JavaScript Exercises: Check if an element is present in the Singly Linked list
JavaScript Singly Linked List: Exercise-18 with Solution
Check if an element is present in a SLL
Write a JavaScript program to check if an element is present in the Singly Linked list.
Sample Solution:
JavaScript Code:
class Node {
// Constructor function for creating a new node with given data
constructor(data) {
this.data = data; // Assign the given data to the node
this.next = null; // Initialize the pointer to the next node as null
}
}
class SinglyLinkedList {
// Constructor function for creating a new singly linked list with optional Head node
constructor(Head = null) {
this.Head = Head; // Initialize the Head node of the list
}
add(newNode){
let node = this.Head; // Start from the Head node
if(node==null){ // If the list is empty
this.Head = newNode; // Set the new node as the Head
return;
}
while (node.next) { // Traverse the list until reaching the last node
node = node.next; // Move to the next node
}
node.next = newNode; // Set the next pointer of the last node to the new node
}
is_present(val) {
let current = this.Head; // Start from the Head node
while (current) { // Iterate through the list
if (current.data === val) { // If the value is found
return true; // Return true indicating presence of value
}
current = current.next; // Move to the next node
}
return false; // Return false indicating absence of value
}
displayList(){
let node = this.Head; // Start from the Head node
var str = "" // Initialize an empty string to store the list elements
while (node) { // Iterate through the list
str += node.data + "->"; // Append the data of the current node to the string
node = node.next; // Move to the next node
}
str += "NULL" // Append "NULL" to indicate the end of the list
console.log(str); // Print the string representing the list
}
}
let numList = new SinglyLinkedList(); // Create a new instance of the SinglyLinkedList class
numList.add(new Node(12)); // Add nodes to the list
numList.add(new Node(13));
numList.add(new Node(14));
numList.add(new Node(15));
numList.add(new Node(14));
numList.add(new Node(16));
console.log("Singly Linked list:"); // Print the original list
numList.displayList();
result = numList.is_present(12); // Check if value 12 is present in the list
console.log("Is 12 present in the said link list: "+result); // Log the result
result = numList.is_present(14); // Check if value 14 is present in the list
console.log("Is 14 present in the said link list: "+result); // Log the result
result = numList.is_present(17); // Check if value 17 is present in the list
console.log("Is 17 present in the said link list: "+result); // Log the result
result = numList.is_present(19); // Check if value 19 is present in the list
console.log("Is 19 present in the said link list: "+result); // Log the result
Output:
Singly Linked list: 12->13->14->15->14->16->NULL Is 12 present in the said link list: true Is 14 present in the said link list: true Is 17 present in the said link list: false Is 19 present in the said link list: false
Flowchart:
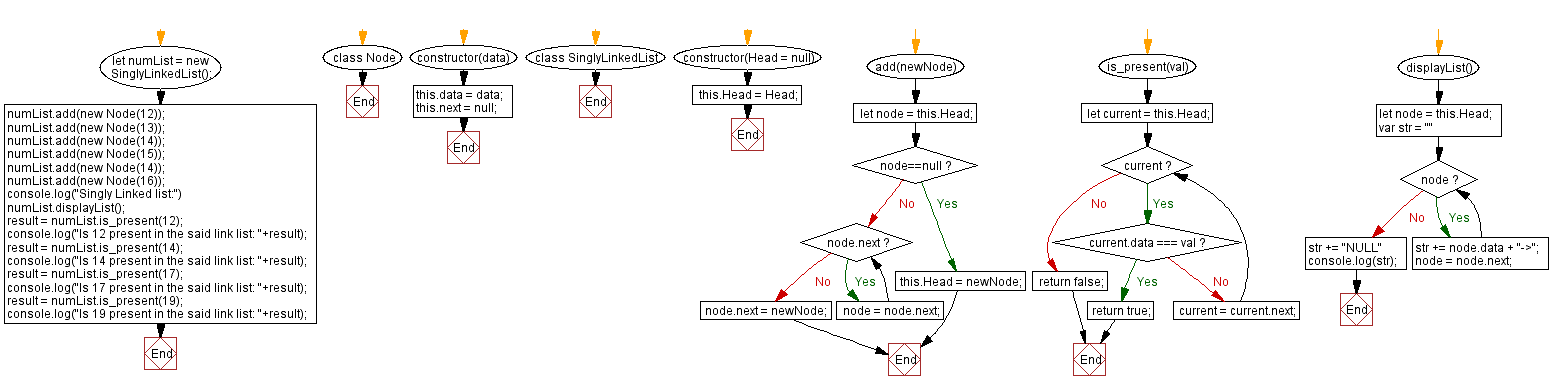
Live Demo:
See the Pen javascript-singly-linked-list-exercise-18 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Singly Linked List Previous: Get index of an given element in a Singly Linked list.
Singly Linked List Next: Create and display Doubly Linked Lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/linkedlist/javascript-singly-linked-list-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics