JavaScript Exercises: Insert a new node at any position of a Singly Linked List
JavaScript Singly Linked List: Exercise-4 with Solution
Insert a node at any position in a SLL
Write a JavaScript program to insert a node at any position in a Singly Linked List.
Sample Solution:
JavaScript Code:
// Define a class representing a node in a singly linked list
class Node {
constructor(data) {
// Initialize the node with provided data
this.data = data
// Initialize the next pointer to null
this.next = null
}
}
// Define a class representing a singly linked list
class SinglyLinkedList {
constructor(Head = null) {
// Initialize the head of the list
this.Head = Head
}
// Method to add a new node to the end of the list
add(newNode){
// Start traversal from the head node
let node = this.Head;
// If the list is empty, set the new node as the head and return
if(node == null){
this.Head = newNode;
return;
}
// Traverse the list until the last node
while (node.next) {
node = node.next;
}
// Set the next pointer of the last node to the new node
node.next = newNode;
}
// Method to insert a new node at a specified index in the list
insertAt(index, newNode){
// Start traversal from the head node
let node = this.Head;
// If the specified index is 0, insert the new node at the beginning of the list
if(index == 0) {
newNode.next = node;
this.head = newNode;
return;
}
// Traverse the list until the node just before the specified index
while(--index){
// If the next node exists, move to the next node
if(node.next !== null)
node = node.next;
// If the next node does not exist and the index is not reached, throw an error
else
throw Error("Index Out of Bound");
}
// Store the next node of the current node in a temporary variable
let tempVal = node.next;
// Set the next pointer of the current node to the new node
node.next = newNode;
// Set the next pointer of the new node to the previously stored next node
newNode.next = tempVal;
}
// Method to display the elements of the list
displayList(){
// Start traversal from the head node
let node = this.Head;
// Initialize an empty string to store the elements of the list
var str = ""
// Traverse the list and concatenate each element to the string
while (node) {
str += node.data + "->";
node = node.next;
}
// Append "NULL" to indicate the end of the list
str += "NULL"
// Print the string containing the list elements
console.log(str);
}
}
// Create an instance of the SinglyLinkedList class
let numList = new SinglyLinkedList();
// Add nodes with data values to the list
numList.add(new Node(12));
numList.add(new Node(13));
numList.add(new Node(14));
numList.add(new Node(15));
// Display the original elements of the list
console.log("Original Linked list:");
numList.displayList();
// Insert a new node with data value 4 at index position 1
console.log("Insert 4 at index position 1:");
numList.insertAt(1, new Node(4));
numList.displayList();
// Insert a new node with data value 5 at index position 3
console.log("Insert 5 at index position 3:");
numList.insertAt(3, new Node(5));
numList.displayList();
// Insert a new node with data value 6 at index position 6
console.log("Insert 6 at index position 6:");
numList.insertAt(6, new Node(6));
numList.displayList();
Output:
12->13->14->15->NULL Insert 4 at index position 1: 12->4->13->14->15->NULL Insert 5 at index position 3: 12->4->13->5->14->15->NULL Insert 6 at index position 6: 12->4->13->5->14->15->6->NULL
Flowchart:
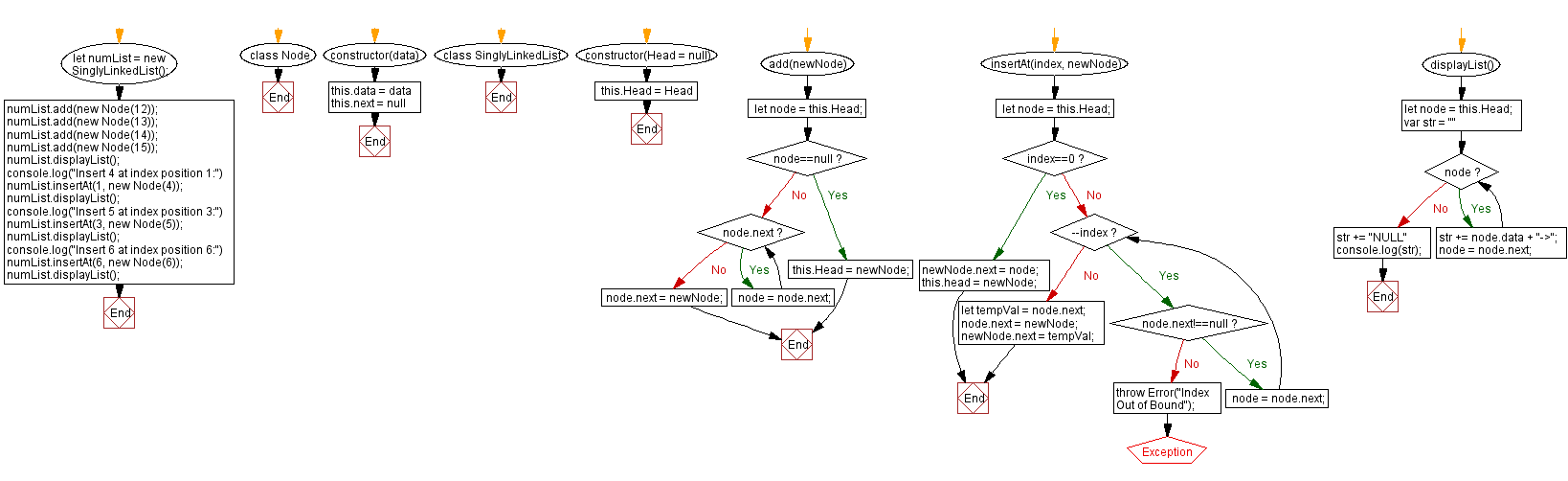
Live Demo:
See the Pen javascript-singly-linked-list-exercise-4 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that inserts a new node at a specified index in a singly linked list and returns the updated list.
- Write a JavaScript function that handles edge cases for insertion at the head, middle, and tail of a singly linked list.
- Write a JavaScript function that inserts a node in a sorted singly linked list, preserving the order.
- Write a JavaScript function that uses recursion to insert a node at the nth position in a singly linked list.
Go to:
PREV : Count the number of nodes in a SLL.
NEXT : Insert a node at the beginning of a SLL.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.