JavaScript Sorting Algorithm: Sort an array of objects, ordered by a property, based on the array of orders provided
JavaScript Sorting Algorithm: Exercise-18 with Solution
Sort Objects by Property Order
Write a JavaScript program to sort an array of objects, ordered by a property, based on the array of orders provided.
- Use Array.prototype.reduce() to create an object from the order array with the values as keys and their original index as the value.
- Use Array.prototype.sort() to sort the given array, skipping elements for which prop is empty or not in the order array.
Sample Solution:
JavaScript Code:
const orderWith = (arr, prop, order) => {
const orderValues = order.reduce((acc, v, i) => {
acc[v] = i;
return acc;
}, {});
return [...arr].sort((a, b) => {
if (orderValues[a[prop]] === undefined) return 1;
if (orderValues[b[prop]] === undefined) return -1;
return orderValues[a[prop]] - orderValues[b[prop]];
});
};
const users = [
{ name: 'fred', language: 'Javascript' },
{ name: 'barney', language: 'TypeScript' },
{ name: 'frannie', language: 'Javascript' },
{ name: 'anna', language: 'Java' },
{ name: 'jimmy' },
{ name: 'nicky', language: 'Python' },
];
console.log(orderWith(users, 'language', ['Javascript', 'TypeScript', 'Java']));
Sample Output:
[{"name":"fred","language":"Javascript"},{"name":"frannie","language":"Javascript"},{"name":"barney","language":"TypeScript"},{"name":"anna","language":"Java"},{"name":"jimmy"},{"name":"nicky","language":"Python"}]
Flowchart:
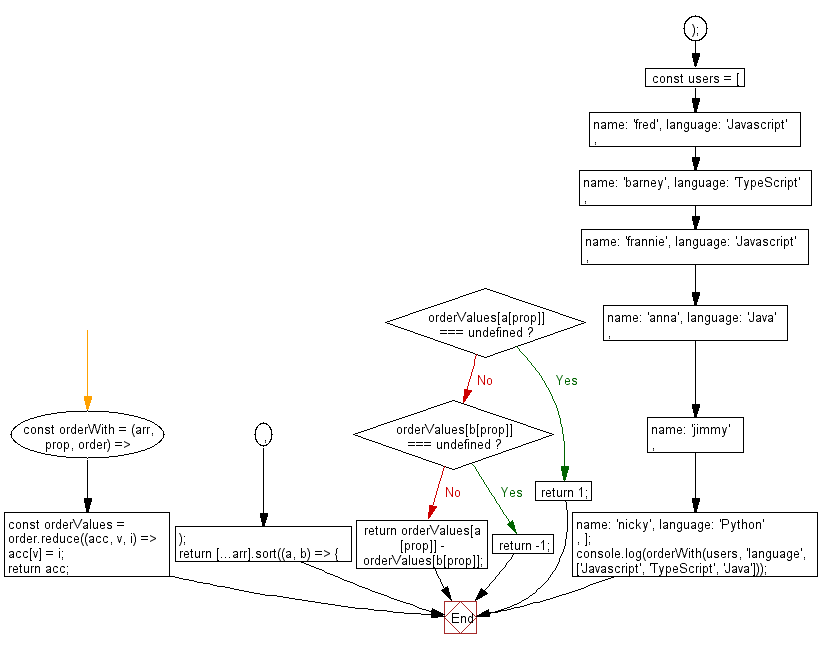
Live Demo:
See the Pen javascript-common-editor by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that sorts an array of objects based on a property, following a specified order provided in another array.
- Write a JavaScript function that reorders objects to match a custom order and places unmatched items at the end.
- Write a JavaScript function that validates the property values against the order array before sorting.
- Write a JavaScript function that logs the sorting process and outputs the sorted object array for verification.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to find the highest index at which a value should be inserted into an array in order to maintain its sort order, based on a provided iterator function.
Next: Write a JavaScript program to sort the characters in a string alphabetically.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.