JavaScript Sorting Algorithm: Alphabetically sorts the characters in a string
JavaScript Sorting Algorithm: Exercise-19 with Solution
Sort Characters in String
Write a JavaScript program to sort the characters in a string alphabetically.
- Use the spread operator (...), Array.prototype.sort() and String.prototype.localeCompare() to sort the characters in str.
- Recombine using String.prototype.join('').
Sample Solution:
JavaScript Code:
const sortCharactersInString = str =>
[...str].sort((a, b) => a.localeCompare(b)).join('');
console.log(sortCharactersInString('cabbage'));
Sample Output:
aabbceg
Flowchart:
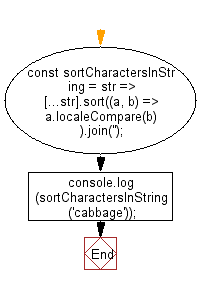
Live Demo:
See the Pen javascript-common-editor by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that sorts the characters of a string alphabetically and returns the new string.
- Write a JavaScript function that converts a string to lowercase, sorts its characters, and then outputs the sorted string.
- Write a JavaScript function that removes non-letter characters before sorting the string's characters.
- Write a JavaScript function that compares the alphabetically sorted string with the original string for a given test case.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to sort an array of objects, ordered by a property, based on the array of orders provided.
Next: Write a JavaScript program to find the lowest index at which a value should be inserted into an array in order to maintain its sorting order, based on the provided iterator function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.