PHP Exercises: Compute the sum of the two given integer values
1. Sum Triple if Same
Write a PHP program to compute the sum of the two given integer values. If the two values are the same, then returns triple their sum.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes two parameters, $x and $y
function test($x, $y)
{
// Use the ternary operator to check if $x is equal to $y
// If true, return the result of multiplying the sum of $x and $y by 3
// If false, return the sum of $x and $y
return $x == $y ? ($x + $y)*3 : $x + $y;
}
// Call the test function with arguments 1 and 2, and echo the result
echo test(1, 2) . "\n";
// Call the test function with arguments 3 and 2, and echo the result
echo test(3, 2) . "\n";
// Call the test function with arguments 2 and 2, and echo the result
echo test(2, 2) . "\n";
?>
Explanation:
- Function Definition:
- A function named test is defined with two parameters: $x and $y.
- Ternary Operation:
- Inside the function, a ternary operator checks if $x is equal to $y.
- If $x == $y, it returns ( $x + $y ) * 3, which is three times the sum of $x and $y.
- If $x is not equal to $y, it returns $x + $y, simply the sum of $x and $y.
- Function Calls and Output:
- First Call: echo test(1, 2);
- Since 1 is not equal to 2, it outputs 1 + 2 = 3.
- Second Call: echo test(3, 2);
- Since 3 is not equal to 2, it outputs 3 + 2 = 5.
- Third Call: echo test(2, 2);
- Since 2 is equal to 2, it outputs (2 + 2) * 3 = 12.
Output:
3 5 12
Visual Presentation:
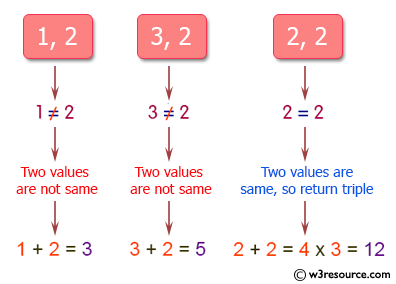
Flowchart:
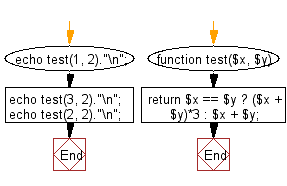
For more Practice: Solve these Related Problems:
- Write a PHP function to compute the sum of two integers and triple the sum if the values are identical, using strict type comparison.
- Write a PHP script to calculate the sum of two integer inputs and, if they match, apply a multiplier by simulating the operation without conditionals.
- Write a PHP program to perform sum computation for two integers, ensuring edge cases with maximum integer values are handled, and triple the sum when both numbers are equal.
- Write a PHP function that uses a ternary operator to check if two integer arguments are the same and then returns triple the sum; otherwise, return their normal sum.
Go to:
PREV : PHP Basic Algorithm Exercises Home
NEXT : Absolute Difference with Triple.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.