PHP Exercises: Get the absolute difference between n and 51
2. Absolute Difference with Triple
Write a PHP program to get the absolute difference between n and 51. If n is greater than 51 return triple the absolute difference.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $n
function test($n)
{
// Declare a variable $x and assign it the value 51
$x = 51;
// Check if $n is greater than $x
if ($n > $x)
{
// If true, return the result of multiplying the difference between $n and $x by 3
return ($n - $x) * 3;
}
// If false, return the difference between $x and $n
return $x - $n;
}
// Call the test function with argument 53 and echo the result
echo test(53) . "\n";
// Call the test function with argument 30 and echo the result
echo test(30) . "\n";
// Call the test function with argument 51 and echo the result
echo test(51) . "\n";
?>
Explanation:
- Function Definition:
- A function named test is defined with a single parameter $n.
- Variable Initialization:
- Inside the function, a variable $x is declared and set to the value 51.
- Conditional Check:
- The function checks if $n is greater than $x (51).
- If $n is greater than $x: It returns ( $n - $x ) * 3, which is three times the difference between $n and $x.
- If $n is not greater than $x: It returns $x - $n, the difference between $x and $n.
- Function Calls and Output:
- First Call: echo test(53);
- Since 53 is greater than 51, it outputs (53 - 51) * 3 = 6.
- Second Call: echo test(30);
- Since 30 is less than 51, it outputs 51 - 30 = 21.
- Third Call: echo test(51);
- Since 51 is equal to 51, it outputs 51 - 51 = 0
Output:
6 21 0
Visual Presentation:
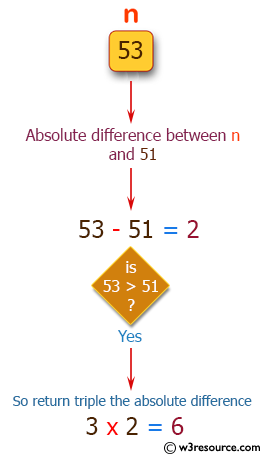
Flowchart:
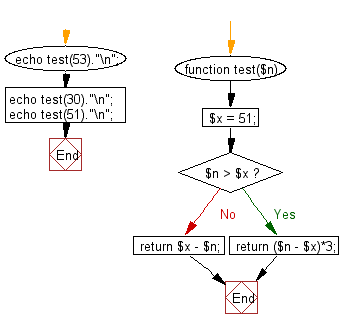
For more Practice: Solve these Related Problems:
- Write a PHP script to compute the absolute difference between a number and 51, and triple the difference if the number exceeds 51, using built-in math functions.
- Write a PHP function that determines the difference from 51 and conditionally multiplies the result by 3 if the input is above 51, while handling negative values.
- Write a PHP program to calculate the absolute difference from 51 and apply a factor of three for any input greater than 51, then test with boundary conditions.
- Write a PHP script to simulate conditional multiplication by 3 on the difference between any number and 51 without using the abs() function.
Go to:
PREV : Sum Triple if Same.
NEXT : Check for 30 or Sum is 30.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.