PHP Exercises: Create an array taking two middle elements from a given array of integers of length even
PHP Basic Algorithm: Exercise-100 with Solution
Write a PHP program to create an array taking two middle elements from a given array of integers of length even.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Calculate the index of the middle element in the array
$middleIndex = sizeof($numbers) / 2;
// Return a new array containing the two elements in the middle of the input array
return [$numbers[$middleIndex - 1], $numbers[$middleIndex]];
}
// Call the 'test' function with an array [1, 5, 7, 9, 11, 13] and store the result in $result
$result = test([1, 5, 7, 9, 11, 13]);
// Print the new array containing the middle two elements using 'implode' and 'echo'
echo "New array: " . implode(',', $result);
?>
Explanation:
- Function Definition:
- A function named test is defined, which takes an array of numbers called $numbers as a parameter.
- Calculate Middle Index:
- The variable $middleIndex is calculated as sizeof($numbers) / 2, which finds the middle index of the array. This calculation assumes the array has an even number of elements.
- Return Middle Elements:
- The function returns an array containing two elements:
- The element just before the middle: $numbers[$middleIndex - 1].
- The element at the middle: $numbers[$middleIndex].
Output:
New array: 7 9
Flowchart:
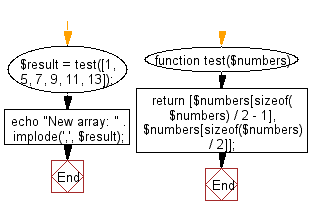
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum.
Next: Write a PHP program to create a new array from two give array of integers, each length 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-100.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics