PHP Exercises: Compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum
99. Sum of Two Arrays and Largest Sum Array
Write a PHP program to compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes two arrays as parameters
function test($nums1, $nums2)
{
// Compare the sum of the first three elements of $nums1 with the sum of the first three elements of $nums2
// If the sum of $nums1 is greater or equal, return $nums1; otherwise, return $nums2
return $nums1[0] + $nums1[1] + $nums1[2] >= $nums2[0] + $nums2[1] + $nums2[2] ? $nums1 : $nums2;
}
// Call the 'test' function with arrays [10, 20, -30] and [10, 20, 30] and store the result in $result
$result = test([10, 20, -30], [10, 20, 30]);
// Print the new array (either $nums1 or $nums2) with maximum values using 'implode' and 'echo'
echo "New array with maximum values: " . implode(',', $result);
?>
Explanation:
- Function Definition:
- A function named test is defined, which takes two arrays as parameters:
- $nums1: the first array of numbers.
- $nums2: the second array of numbers.
- Compare Sums of Elements:
- The function calculates the sum of the first three elements in each array:
- Sum of $nums1: $nums1[0] + $nums1[1] + $nums1[2].
- Sum of $nums2: $nums2[0] + $nums2[1] + $nums2[2].
- It then compares these sums:
- If the sum of $nums1 is greater than or equal to the sum of $nums2, the function returns $nums1.
- Otherwise, it returns $nums2.
- Test Case:
- The test function is called with two arrays: [10, 20, -30] and [10, 20, 30].
- The result is stored in $result.
- Display the Result:
- The resulting array (either $nums1 or $nums2) is printed.
Output:
New array with maximum values: 10,20,30
Flowchart:
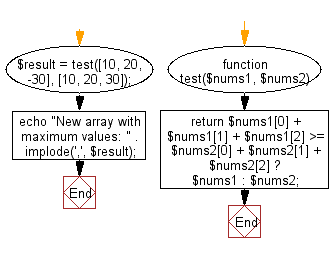
For more Practice: Solve these Related Problems:
- Write a PHP script to compute the sum of two three-element arrays and then return the array that has the greater total sum.
- Write a PHP function to add two fixed arrays element-wise and compare their overall sums to determine the one with the larger value.
- Write a PHP program to calculate sums for two arrays and output the array corresponding to the highest cumulative value.
- Write a PHP script to compare the totals of two three-number arrays and display the array with the maximum sum.
Go to:
PREV : Replace 7 with 1 Following 5 in Array.
NEXT : Array of Middle Two Elements (Even Length).
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.