PHP Exercises: Create a new array swapping the first and last elements of a given array of integers and length will be least 1
102. Swap First and Last Elements in Array
Write a PHP program to create a new array swapping the first and last elements of a given array of integers and length will be least 1.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Store the value of the first element in a variable named $first
$first = $numbers[0];
// Swap the value of the first element with the value of the last element in the array
$numbers[0] = $numbers[sizeof($numbers) - 1];
// Set the value of the last element to the stored value of the first element
$numbers[sizeof($numbers) - 1] = $first;
// Return the modified array after swapping the first and last elements
return $numbers;
}
// Call the 'test' function with an array [1, 5, 7, 9, 11, 13] and store the result in $result
$result = test([1, 5, 7, 9, 11, 13]);
// Print the new array after swapping the first and last elements using 'implode' and 'echo'
echo "New array, after swapping first and last elements: " . implode(',', $result);
?>
Sample Output:
New array, after swapping first and last elements: 13,5,7,9,11,1
Flowchart:
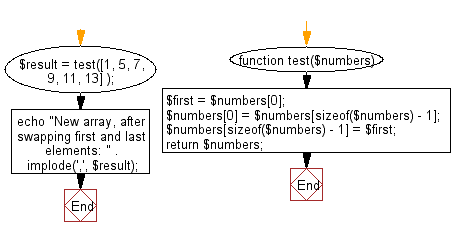
For more Practice: Solve these Related Problems:
- Write a PHP script to swap the first and last elements of an array and output the updated array.
- Write a PHP function that exchanges the boundary elements of an array and preserves the order of the middle elements.
- Write a PHP program to perform an in-place swap of the first and last elements using temporary variables.
- Write a PHP script to validate an array’s length and then swap its initial and final items, using array indices.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new array from two give array of integers, each length 3.
Next: Write a PHP program to create a new array length 3 from a given array (length atleast 3) using the elements from the middle of the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.