PHP Exercises: Create a new array length 3 from a given array using the elements from the middle of the array
PHP Basic Algorithm: Exercise-103 with Solution
Write a PHP program to create a new array length 3 from a given array (length atleast 3) using the elements from the middle of the array.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Calculate the index of the middle element in the array
$middleIndex = sizeof($numbers) / 2;
// Return a new array containing the three elements around the middle of the input array
return [$numbers[$middleIndex - 1], $numbers[$middleIndex], $numbers[$middleIndex + 1]];
}
// Call the 'test' function with an array [1, 5, 7, 9, 11, 13] and store the result in $result
$result = test([1, 5, 7, 9, 11, 13]);
// Print the new array containing the three elements around the middle using 'implode' and 'echo'
echo "New array: " . implode(',', $result);
?>
Sample Output:
New array: 7,9,11
Flowchart:
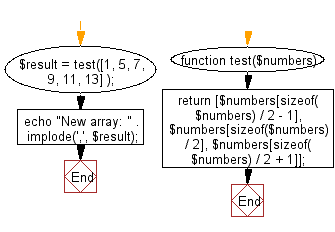
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new array from two give array of integers, each length 3.
Next: Write a PHP program to find the largest value from first, last, and middle elements of a given array of integers of odd length (atleast 1).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-103.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics