PHP Exercises: Find the largest value from first, last, and middle elements of a given array of integers of odd length
PHP Basic Algorithm: Exercise-104 with Solution
Write a PHP program to find the largest value from first, last, and middle elements of a given array of integers of odd length (atleast 1).
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Store the value of the first element in a variable named $first
$first = $numbers[0];
// Store the value of the middle element in a variable named $middle_ele
$middle_ele = $numbers[sizeof($numbers) / 2];
// Store the value of the last element in a variable named $last_ele
$last_ele = $numbers[sizeof($numbers) - 1];
// Initialize a variable named $max_ele with the value of $first
$max_ele = $first;
// Check if $middle_ele is greater than $max_ele, update $max_ele if true
if ($middle_ele > $max_ele)
{
$max_ele = $middle_ele;
}
// Check if $last_ele is greater than $max_ele, update $max_ele if true
if ($last_ele > $max_ele)
{
$max_ele = $last_ele;
}
// Return the maximum value among the three elements
return $max_ele;
}
// Call the 'test' function with different arrays and print the results
echo test([1]) . "\n";
echo test([1,2,9]) . "\n";
echo test([1,2,9,3,3]) . "\n";
echo test([1,2,3,4,5,6,7]) . "\n";
echo test([1,2,2,3,7,8,9,10,6,5,4]) . "\n";
?>
Sample Output:
1 9 9 7 8
Flowchart:
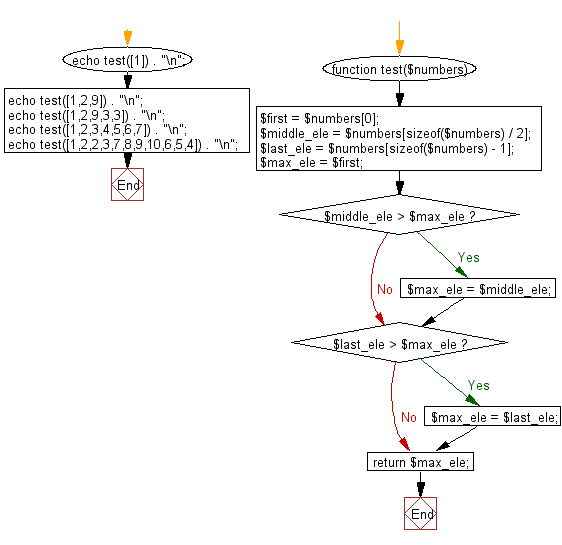
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new array length 3 from a given array (length atleast 3) using the elements from the middle of the array.
Next: Write a PHP program to create a new array taking the first two elements from a given array. If the length of the given array is less than 2 then return the give array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-104.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics