PHP Exercises: Create a new array taking the first two elements from a given array
105. New Array from First Two Elements
Write a PHP program to create a new array taking the first two elements from a given array. If the length of the given array is less than 2 then return the give array.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Check if the size of the array is greater than or equal to 2
if (sizeof($numbers) >= 2)
{
// If true, create a new array $front_nums with the first two elements of $numbers
$front_nums = [$numbers[0], $numbers[1]];
}
// Check if the size of the array is greater than 0 (but less than 2)
else if (sizeof($numbers) > 0)
{
// If true, create a new array $front_nums with the first element of $numbers
$front_nums = [$numbers[0]];
}
// If the array is empty
else
{
// Create an empty array $front_nums
$front_nums = [];
}
// Return the array $front_nums
return $front_nums;
}
// Call the 'test' function with an array [1, 5, 7, 9, 11, 13] and store the result in $result
$result = test([1, 5, 7, 9, 11, 13]);
// Print the new array $front_nums using 'implode' and 'echo'
echo "New array: " . implode(',', $result);
?>
Sample Output:
New array: 1,5
Flowchart:
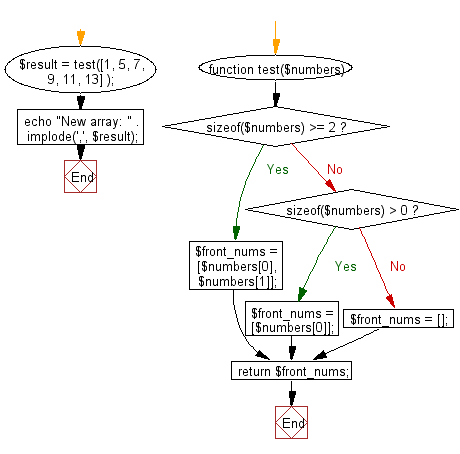
For more Practice: Solve these Related Problems:
- Write a PHP script to extract the first two elements of an array and output them as a new array.
- Write a PHP function that checks the length of an array and returns its first two items if available.
- Write a PHP program to slice an array to produce a new array containing only its first two elements.
- Write a PHP script to handle edge cases by returning the entire array if its length is less than two, otherwise outputting the first two elements.
Go to:
PREV : Largest from First, Last, and Middle.
NEXT : Count Even Numbers in Array.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.