PHP Exercises: Create a new string taking the first 3 characters of a given string and return the string with the 3 characters added at both the front and back
11. Add First 3 Characters Front and Back
Write a PHP program to create a new string taking the first 3 characters of a given string and return the string with the 3 characters added at both the front and back. If the given string length is less than 3, use whatever characters are there.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $str
function test($str)
{
// Use an if-else statement to check if the length of $str is less than 3
if (strlen($str) < 3) {
// If true, concatenate $str with itself twice and return the result
return $str . $str . $str;
} else {
// If false, use substr to get the first three characters of $str
$front = substr($str, 0, 3);
// Concatenate $front, $str, and $front, then return the result
return $front . $str . $front;
}
}
// Call the test function with argument "Python" and echo the result
echo test("Python") . "\n";
// Call the test function with argument "JS" and echo the result
echo test("JS") . "\n";
// Call the test function with argument "Code" and echo the result
echo test("Code") . "\n";
?>
Explanation:
- Function Definition:
- The test function takes one parameter, $str.
- Length Check:
- If the length of $str is less than 3 characters:
- Concatenate $str with itself twice (creating a string of three copies) and return this result.
- If $str has 3 or more characters:
- Extract the first three characters of $str and store it in $front.
- Concatenate $front, $str, and $front again, and return this new string.
- Function Calls and Output:
- First Call: test("Python")
- $str has more than 3 characters, so $front is "Pyt".
- Returns "PytPythonPyt".
- Second Call: test("JS")
- $str has fewer than 3 characters, so returns "JSJSJS".
- Third Call: test("Code")
- $str has more than 3 characters, so $front is "Cod".
- Returns "CodCodeCod".
Output:
PytPythonPyt JSJSJS CodCodeCod
Visual Presentation:
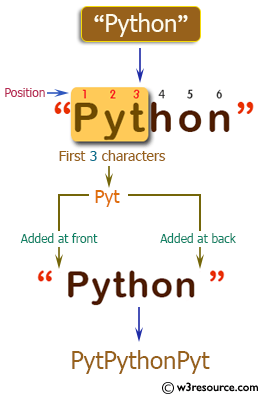
Flowchart:
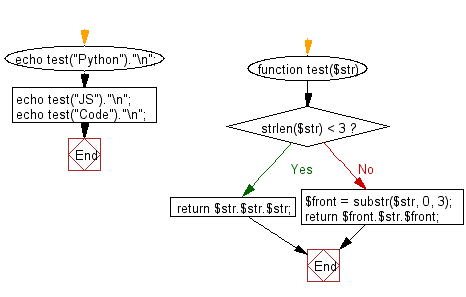
For more Practice: Solve these Related Problems:
- Write a PHP script to form a new string by adding the first three characters of the input string to both its beginning and end, handling short strings appropriately.
- Write a PHP function that takes a string and, if its length is less than three, repeats the entire string as a prefix and suffix.
- Write a PHP program to build a new string by extracting the first three characters and appending them to the front and back of the original string.
- Write a PHP script to replicate the first three characters as both a header and trailer for the given string using concatenation.
Go to:
PREV : Check Multiple of 3 or 7.
NEXT : Check if String Starts with "C#".
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.