PHP Exercises: Check if a given positive number is a multiple of 3 or a multiple of 7
10. Check Multiple of 3 or 7
Write a PHP program to check if a given positive number is a multiple of 3 or a multiple of 7.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $n
function test($n)
{
// Use the modulo operator to check if $n is divisible by 3 or 7
return $n % 3 == 0 || $n % 7 == 0;
}
// Call the test function with argument 3 and var_dump the result
var_dump(test(3));
// Call the test function with argument 14 and var_dump the result
var_dump(test(14));
// Call the test function with argument 12 and var_dump the result
var_dump(test(12));
// Call the test function with argument 37 and var_dump the result
var_dump(test(37));
?>
Explanation:
- Function Definition:
- The function test takes one parameter, $n.
- Divisibility Check:
- Uses the modulo operator % to check if $n is divisible by either 3 or 7.
- Returns true if $n is divisible by 3 ($n % 3 == 0) or by 7 ($n % 7 == 0); otherwise, returns false.
- Function Calls and Output:
- First Call: test(3)
- 3 % 3 == 0, so it returns true.
- Second Call: test(14)
- 14 % 7 == 0, so it returns true.
- Third Call: test(12)
- 12 % 3 == 0, so it returns true.
- Fourth Call: test(37)
- 37 is not divisible by either 3 or 7, so it returns false.
Output:
bool(true) bool(true) bool(true) bool(false)
Visual Presentation:
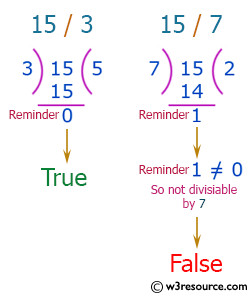
Flowchart:
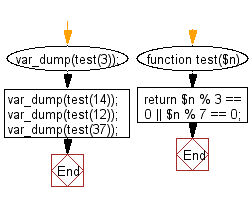
For more Practice: Solve these Related Problems:
- Write a PHP script to check if a positive number is divisible by 3 or 7, using modulus operators and proper condition grouping.
- Write a PHP function to validate divisibility by 3 and 7 separately and then combine the results with logical operators.
- Write a PHP program to determine the divisibility of an integer by 3 or 7 without using modulus operators.
- Write a PHP script to check the factors of a given number and return true if it is a multiple of either 3 or 7, otherwise false.
Go to:
PREV : Add Last Character at Front and Back.
NEXT : Add First 3 Characters Front and Back.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.