PHP Exercises: Create a new string with the last char added at the front and back of a given string of length 1 or more
PHP Basic Algorithm: Exercise-9 with Solution
Write a PHP program to create a new string with the last char added at the front and back of a given string of length 1 or more.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $str
function test($str)
{
// Use the substr function to get the last character of $str
$s = substr($str, strlen($str) - 1);
// Concatenate the last character, $str, and the last character again, then return the result
return $s . $str . $s;
}
// Call the test function with argument "Red" and echo the result
echo test("Red") . "\n";
// Call the test function with argument "Green" and echo the result
echo test("Green") . "\n";
// Call the test function with argument "1" and echo the result
echo test("1") . "\n";
?>
Explanation:
- Function Definition:
- The function test takes one parameter, $str.
- Extracting the Last Character:
- Uses substr($str, strlen($str) - 1) to get the last character of $str and assigns it to $s.
- Concatenation:
- Concatenates $s (last character), $str (the original string), and $s (last character again) to create a new string with the last character at both the beginning and end.
- Returns this concatenated result.
- Function Calls and Output:
- First Call: test("Red")
- Last character is "d", so the result is "dRedd".
- Second Call: test("Green")
- Last character is "n", so the result is "nGreenn".
- Third Call: test("1")
- Last character is "1" (the only character), so the result is "111".
Output:
dRedd nGreenn 111
Visual Presentation:
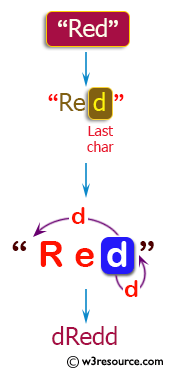
Flowchart:
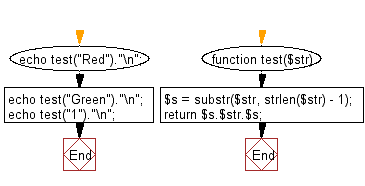
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string which is 4 copies of the 2 front characters of a given string. If the given string length is less than 2 return the original string.
Next:Write a PHP program to check if a given positive number is a multiple of 3 or a multiple of 7.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics