PHP Exercises: Check if a given array of integers contains 5 next to a 5 somewhere
110. Contains 5 Next to 5 Check in Array
Write a PHP program to check if a given array of integers contains 5 next to a 5 somewhere.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Iterate through the elements of the array up to the second-to-last element
for ($i = 0; $i < sizeof($nums) - 1; $i++)
{
// Check if the current element is 5 and is equal to the next element
if ($nums[$i] == 5 && $nums[$i] == $nums[$i + 1]) return true;
}
// Return false if there are no consecutive elements equal to 5
return false;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([1, 5, 6, 9, 10, 17]));
var_dump(test([1, 5, 5, 9, 10, 17]));
var_dump(test([1, 5, 5, 9, 10, 17, 5, 5]));
?>
Sample Output:
bool(false) bool(true) bool(true)
Flowchart:
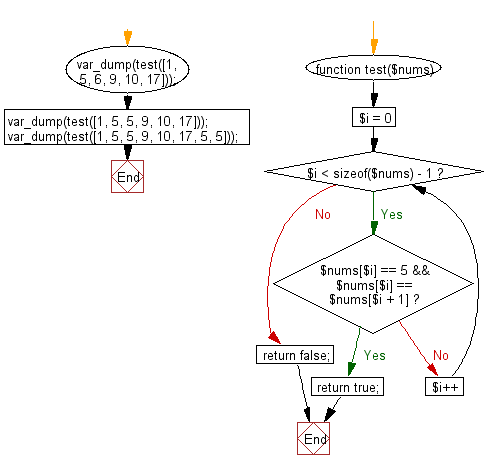
For more Practice: Solve these Related Problems:
- Write a PHP script to scan an array of integers and return true if any two adjacent elements are both 5.
- Write a PHP function to iterate through an array and check for consecutive occurrences of the number 5.
- Write a PHP program to use a loop to compare neighboring elements and verify the presence of a pair of 5's.
- Write a PHP script to determine if the given array contains a 5 immediately followed by another 5 using index-based comparisons.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compute the sum of the numbers in a given array except those numbers starting with 5 followed by atleast one 6. Return 0 if the given array has no integer.
Next: Write a PHP program to check whether a given array of integers contains 5's and 7's
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.