PHP Exercises: Check whether a given array of integers contains 5's and 7's
111. Contains Both 5 and 7 in Array
Write a PHP program to check whether a given array of integers contains 5's and 7's.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($nums); $i++)
{
// Check if the current element is equal to 5 or 7
if ($nums[$i] == 5 || $nums[$i] == 7) return true;
}
// Return false if there are no elements equal to 5 or 7
return false;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([1, 5, 6, 9, 10, 17]));
var_dump(test([1, 4, 7, 9, 10, 17]));
var_dump(test([1, 1, 2, 9, 10, 17]));
Sample Output:
bool(true) bool(true) bool(false)
Flowchart:
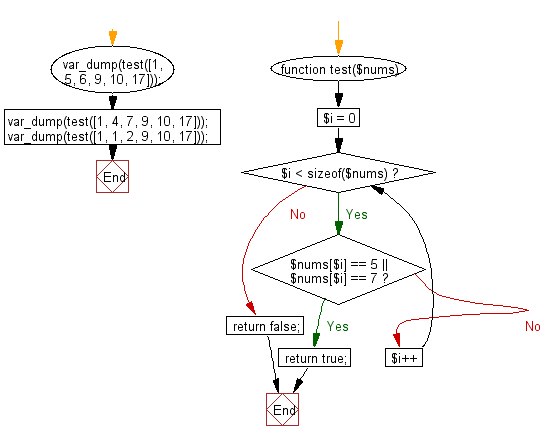
For more Practice: Solve these Related Problems:
- Write a PHP script to check if an array contains at least one occurrence of both 5 and 7.
- Write a PHP function to scan an array and return true if the set of elements includes both 5 and 7.
- Write a PHP program to implement array searching logic to detect the presence of the numbers 5 and 7 simultaneously.
- Write a PHP script to iterate through an array, use flags for both 5 and 7, and output a boolean if both exist.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check if a given array of integers contains 5 next to a 5 somewhere.
Next: Write a PHP program to check if the sum of all 5' in the array exactly 15 in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.