PHP Exercises: Check if the sum of all 5' in the array exactly 15 in a given array of integers
112. Sum of 5's Equals 15 Check
Write a PHP program to check if the sum of all 5' in the array exactly 15 in a given array of integers.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Initialize a variable $sum with a value of 0
$sum = 0;
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($nums); $i++)
{
// Check if the current element is equal to 5, increment $sum by 5 if true
if ($nums[$i] == 5)
$sum += 5;
}
// Return true if the sum is equal to 15, otherwise return false
return $sum == 15;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([1, 5, 6, 9, 10, 17]));
var_dump(test([1, 5, 5, 5, 10, 17]));
var_dump(test([1, 1, 5, 5, 5, 5]));
?>
Sample Output:
bool(false) bool(true) bool(false)
Flowchart:
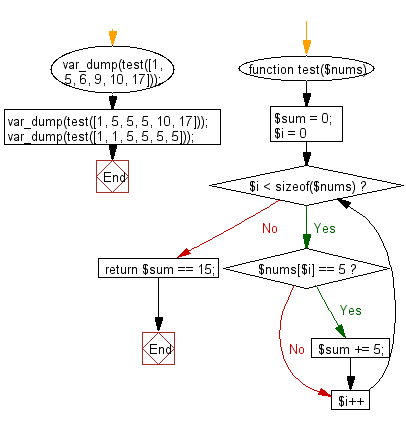
For more Practice: Solve these Related Problems:
- Write a PHP script to calculate the sum of occurrences of the number 5 in an array and return true if the sum equals 15.
- Write a PHP function to count how many times 5 appears in an array and check if that count multiplied by 5 equals 15.
- Write a PHP program to iterate over an array, sum only the elements that are 5, and compare the total with 15.
- Write a PHP script to use array filtering to collect all 5's then sum them and check for the target sum of 15.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check whether a given array of integers contains 5's and 7's.
Next: Write a PHP program to check if the number of 3's is greater than the number of 5's.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.