PHP Exercises: Check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array
118. Array Contains 3 Followed Later by 5
Write a PHP program to check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Initialize a variable $three with a value of false
$three = false;
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($numbers); $i++)
{
// Check if both $three is true and the current element is equal to 5, return true if true
if ($three && $numbers[$i] == 5) return true;
// Check if the current element is equal to 3, set $three to true if true
if ($numbers[$i] == 3) $three = true;
}
// Return false if there are no occurrences of 3 followed by 5 in the array
return false;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([3, 5, 1, 3, 7]));
var_dump(test([1, 2, 3, 4]));
var_dump(test([3, 3, 5, 5, 5, 5]));
var_dump(test([2, 5, 5, 7, 8, 10]));
?>
Sample Output:
bool(true) bool(false) bool(true) bool(false)
Flowchart:
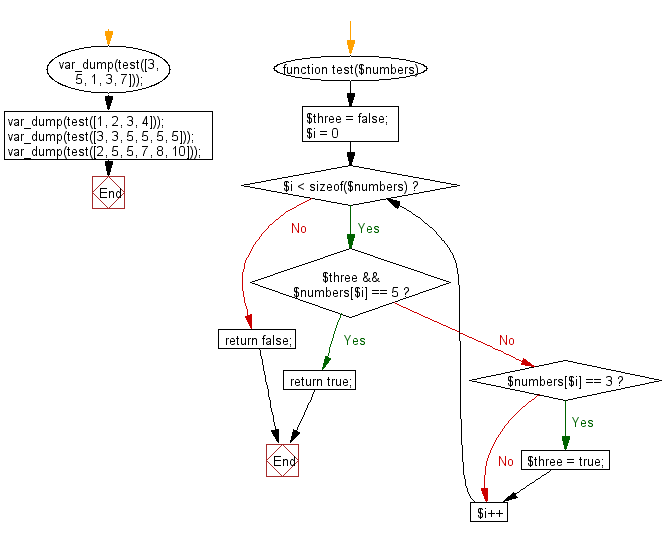
For more Practice: Solve these Related Problems:
- Write a PHP script to scan an array and return true if a 3 appears before a 5, regardless of the gap between them.
- Write a PHP function to check if the first occurrence of 3 in an array is followed at any later point by a 5.
- Write a PHP program to iterate over an array and use flags to indicate whether a 3 is encountered before any subsequent 5.
- Write a PHP script to implement logic that sets a marker upon encountering a 3 and then verifies if a 5 appears later in the array.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check a given array of integers and return true if the given array contains two 5's next to each other, or two 5 separated by one element.
Next: Write a PHP program to check a given array of integers and return true if the given array contains either 2 even or 2 odd values all next to each other.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.