PHP Exercises: Check a given array of integers and return true if the value 5 appears 5 times and there are no 5 next to each other
120. Check for Five Occurrences of 5 Without Neighbors
Write a PHP program to check a given array of integers and return true if the value 5 appears 5 times and there are no 5 next to each other.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Initialize variables $flag and $five with values of false and 0, respectively
$flag = false;
$five = 0;
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($numbers); $i++)
{
// Check if the current element is 5 and $flag is false
if ($numbers[$i] == 5 && !$flag)
{
// Increment $five and set $flag to true if the condition is met
$five++;
$flag = true;
}
else
{
// Set $flag to false if the current element is not 5
$flag = false;
}
}
// Return true if there are five consecutive occurrences of 5, otherwise false
return $five == 5;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([3, 5, 1, 5, 3, 5, 7, 5, 1, 5]));
var_dump(test([3, 5, 5, 5, 5, 5, 5]));
var_dump(test([3, 5, 2, 5, 4, 5, 7, 5, 8, 5]));
var_dump(test([2, 4, 5, 5, 5, 5]));
?>
Sample Output:
bool(true) bool(false) bool(true) bool(false)
Flowchart:
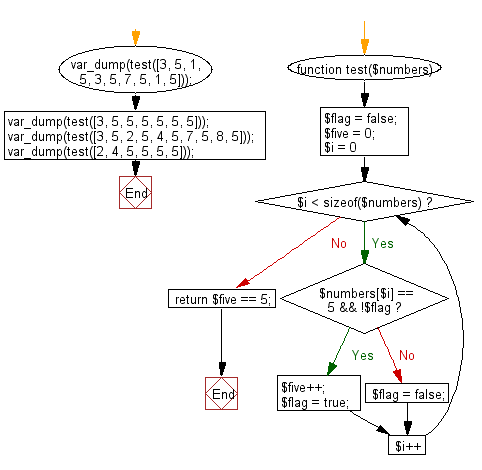
For more Practice: Solve these Related Problems:
- Write a PHP script to examine an array and return true if the number 5 appears exactly five times without any two 5’s being adjacent.
- Write a PHP function to count occurrences of 5 and ensure that no 5 is immediately adjacent to another 5.
- Write a PHP program to iterate over an array and use conditional logic to ensure exactly five isolated 5's exist.
- Write a PHP script to scan an array for 5's while verifying that every 5 is separated by at least one different number, and return a boolean.
Go to:
PREV : Check for Two Consecutive Even or Odd Values.
NEXT : Check Every 5 is Paired with Another.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.