PHP Exercises: Check if one given temperatures is less than 0 and the other is greater than 100
13. Temperature Extremes Check
Write a PHP program to check if one given temperatures is less than 0 and the other is greater than 100.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes two parameters: $temp1 and $temp2
function test($temp1, $temp2)
{
// Check if $temp1 is less than 0 and $temp2 is greater than 100,
// or if $temp2 is less than 0 and $temp1 is greater than 100.
return $temp1 < 0 && $temp2 > 100 || $temp2 < 0 && $temp1 > 100;
}
// Call the test function with arguments 120 and -1, and var_dump the result
var_dump(test(120, -1));
// Call the test function with arguments -1 and 120, and var_dump the result
var_dump(test(-1, 120));
// Call the test function with arguments 2 and 120, and var_dump the result
var_dump(test(2, 120));
?>
Explanation:
- Function Definition:
- The test function accepts two parameters, $temp1 and $temp2.
- Condition Check:
- The function checks if either of the following conditions is true:
- $temp1 is less than 0, and $temp2 is greater than 100.
- $temp2 is less than 0, and $temp1 is greater than 100.
- If either condition is met, the function returns true; otherwise, it returns false.
- Function Calls and Output:
- First Call: test(120, -1)
- $temp1 is greater than 100 and $temp2 is less than 0, so it returns true.
- Second Call: test(-1, 120)
- $temp1 is less than 0 and $temp2 is greater than 100, so it returns true.
- Third Call: test(2, 120)
- Neither condition is met, so it returns false.
Output:
bool(true) bool(true) bool(false)
Visual Presentation:
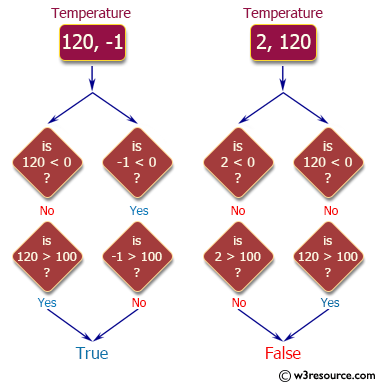
Flowchart:
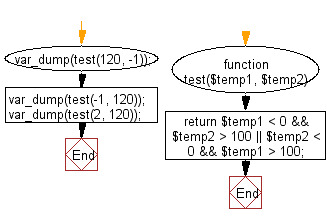
For more Practice: Solve these Related Problems:
- Write a PHP script to determine if one temperature is below 0 and the other above 100 using relational operators.
- Write a PHP function to check two temperature values and return true if one is negative while the other exceeds 100.
- Write a PHP program to validate temperature extremes where one value is below freezing and the other is above boiling point.
- Write a PHP script to compare two temperatures and output a boolean based on whether they cross the specified extreme thresholds.
Go to:
PREV : Check if String Starts with "C#".
NEXT : Integers in Range 100..200.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.