PHP Exercises: Check two given integers whether either of them is in the range 100..200 inclusive
14. Integers in Range 100..200
Write a PHP program to check two given integers whether either of them is in the range 100..200 inclusive.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes two parameters: $x and $y
function test($x, $y)
{
// Check if $x is between 100 and 200 (inclusive) or if $y is between 100 and 200 (inclusive)
return ($x >= 100 && $x <= 200) || ($y >= 100 && $y <= 200);
}
// Call the test function with arguments 100 and 199, and var_dump the result
var_dump(test(100, 199));
// Call the test function with arguments 250 and 300, and var_dump the result
var_dump(test(250, 300));
// Call the test function with arguments 105 and 190, and var_dump the result
var_dump(test(105, 190));
?>
Explanation:
- Function Definition:
- The test function takes two parameters: $x and $y.
- Condition Check:
- The function checks if:
- $x is between 100 and 200 (inclusive), or
- $y is between 100 and 200 (inclusive).
- If either $x or $y meets this condition, the function returns true; otherwise, it returns false.
- Function Calls and Output:
- First Call: test(100, 199)
- $x is 100, which is within the range [100, 200], so it returns true.
- Second Call: test(250, 300)
- Neither $x nor $y is within the range [100, 200], so it returns false.
- Third Call: test(105, 190)
- Both $x and $y are within the range [100, 200], so it returns true.
- First Call: test(100, 199)
Output:
bool(true) bool(false) bool(true)
Visual Presentation:
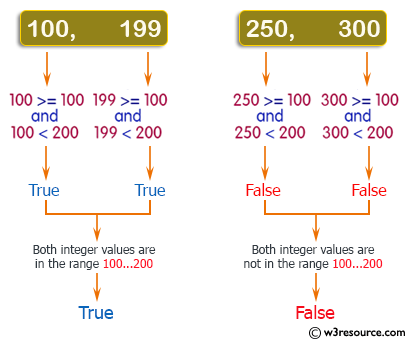
Flowchart:
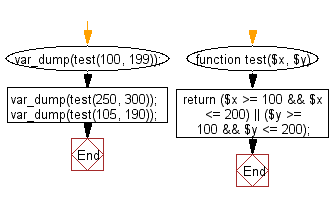
For more Practice: Solve these Related Problems:
- Write a PHP script to test two integers to determine if at least one falls within the range of 100 to 200 inclusive.
- Write a PHP function to validate if either of two numbers is between 100 and 200 using conditional checks.
- Write a PHP program to check for boundary compliance of two numbers and return a boolean if one is within the specified range.
- Write a PHP script to compare two integer inputs and use logical operators to output true if one is within 100 to 200.
Go to:
PREV : Temperature Extremes Check.
NEXT : Three Integers in Range 20..50.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.