PHP Exercises: Check a given array of integers and return true if there are two values 15, 15 next to each other
131. Check for Two Adjacent 15's in Array
Write a PHP program to check a given array (length will be atleast 2) of integers and return true if there are two values 15, 15 next to each other.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Iterate through the elements of the input array using a for loop
for ($i = 0; $i < sizeof($numbers) - 1; $i++)
{
// Check if the next element is equal to the current element, and both are equal to 15
if ($numbers[$i + 1] == $numbers[$i] && $numbers[$i] == 15)
{
// If true, return true, indicating the presence of consecutive 15s
return true;
}
}
// If the loop completes without finding consecutive 15s, return false
return false;
}
// Use the 'var_dump' function to print the result of the 'test' function for different arrays
var_dump(test([5, 5, 1, 15, 15]));
var_dump(test([15, 2, 3, 4, 15]));
var_dump(test([3, 3, 15, 15, 5, 5]));
var_dump(test([1, 5, 15, 7, 8, 15]));
?>
Sample Output:
bool(true) bool(false) bool(true) bool(false)
Flowchart:
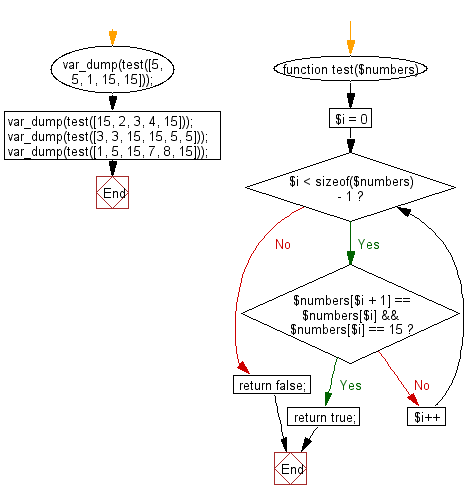
For more Practice: Solve these Related Problems:
- Write a PHP script to check if a given array of integers contains two consecutive values of 15.
- Write a PHP function to scan an array for adjacent pairs that equal 15 and return true if found.
- Write a PHP program to iterate over an array and detect if any two neighboring elements are both 15.
- Write a PHP script to use index comparison to verify the presence of 15 appearing twice in a row in an array.
Go to:
PREV : Check Non-Decreasing Order of Array.
NEXT : Larger Average between First and Second Halves.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.