PHP Exercises: Find the larger average value between the first and the second half of a given array of integers and minimum length is atleast 2
132. Larger Average between First and Second Halves
Write a PHP program to find the larger average value between the first and the second half of a given array of integers and minimum length is atleast 2. Assume that the second half begins at index (array length)/2.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Calculate the average of the first half of the array using the 'Average' function
$firstHalf = Average($numbers, 0, sizeof($numbers) / 2);
// Calculate the average of the second half of the array using the 'Average' function
$secondHalf = Average($numbers, sizeof($numbers) / 2, sizeof($numbers));
// Return the larger of the two averages
return $firstHalf > $secondHalf ? $firstHalf : $secondHalf;
}
// Define a function named 'Average' that calculates the average of a specified range within an array
function Average($num, $start, $end)
{
// Initialize a variable to store the sum of elements in the specified range
$sum = 0;
// Iterate through the specified range and calculate the sum of elements
for ($i = $start; $i < $end; $i++)
$sum += $num[$i];
// Return the average of the specified range
return $sum / (sizeof($num) / 2);
}
// Use the 'echo' statement to print the result of the 'test' function for different arrays
echo test([1, 2, 3, 4, 6, 8]) . "\n";
echo test([15, 2, 3, 4, 15, 11]) . "\n";
?>
Sample Output:
6 10
Flowchart:
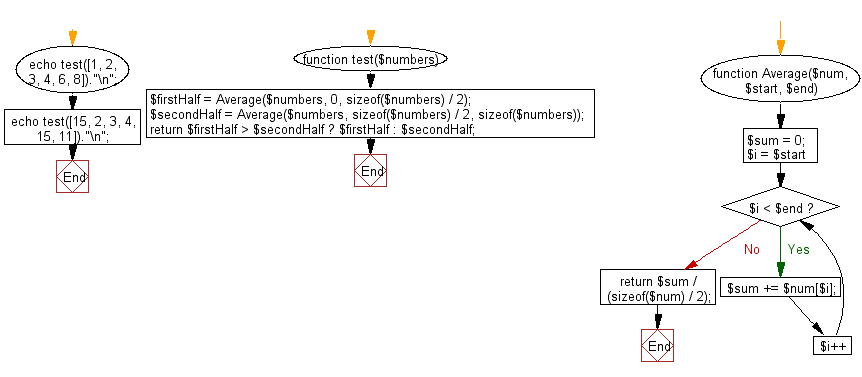
For more Practice: Solve these Related Problems:
- Write a PHP script to divide an array into two halves and compare the averages of each half, returning the larger average.
- Write a PHP function to split an even-length array at the midpoint and compute the average of each segment, then output the greater one.
- Write a PHP program to calculate the mean of the first and second halves of an array and determine which average is higher.
- Write a PHP script to process an array by computing two averages and returning the maximum of the two values.
Go to:
PREV : Check for Two Adjacent 15's in Array.
NEXT : Count Strings of Given Length in Array.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.