PHP Exercises: Count the number of strings with given length in given array of strings
133. Count Strings of Given Length in Array
Write a PHP program to count the number of strings with given length in given array of strings.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of strings and a length parameter
function test($arr_str, $len)
{
// Initialize a counter variable to keep track of the number of strings with the specified length
$ctr = 0;
// Iterate through the array of strings
for ($i = 0; $i < sizeof($arr_str); $i++)
{
// Check if the length of the current string matches the specified length
if (strlen($arr_str[$i]) == $len)
$ctr++; // Increment the counter if the length matches
}
// Return the final count of strings with the specified length
return $ctr;
}
// Use the 'echo' statement to print the result of the 'test' function for the given array and length
echo test(["a", "b", "bb", "c", "ccc"], 1) . "\n";
?>
Sample Output:
3
Flowchart:
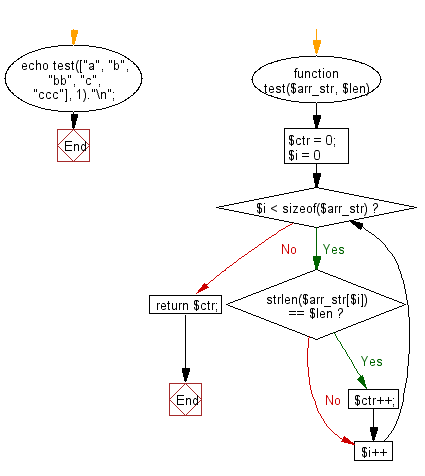
For more Practice: Solve these Related Problems:
- Write a PHP script to iterate over an array of strings and count how many have a specified length.
- Write a PHP function to filter an array of strings based on a given length and return the count of matches.
- Write a PHP program to use strlen() in a loop to tally the number of strings that match a particular length requirement.
- Write a PHP script to employ array filtering to count strings with a given character count from an input array.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to find the larger average value between the first and the second half of a given array of integers and minimum length is atleast 2. Assume that the second half begins at index (array length)/2.
Next: Write a PHP program to create a new array using the first n strings from a given array of strings. (n>=1 and <=length of the array).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.