PHP Exercises: Create a new array using the first n strings from a given array of strings
134. New Array from First n Strings
Write a PHP program to create a new array using the first n strings from a given array of strings. (n>=1 and <=length of the array).
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of strings and an integer 'n'
function test($arr_str, $n)
{
// Initialize a new array with a size equal to the number of elements in the input array
$new_array = [sizeof($arr_str)];
// Iterate through the array up to the specified 'n' elements
for ($i = 0; $i < $n; $i++)
{
// Copy each element from the input array to the new array
$new_array[$i] = $arr_str[$i];
}
// Return the new array containing the first 'n' elements
return $new_array;
}
// Call the 'test' function with an array and 'n' value, then use 'implode' to print the result as a string
$result = test(["a", "b", "bb", "c", "ccc"], 3);
echo implode(" ", $result);
?>
Sample Output:
a b bb
Flowchart:
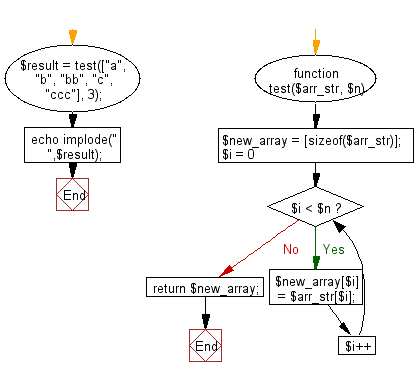
For more Practice: Solve these Related Problems:
- Write a PHP script to take the first n elements from an array of strings and output a new array containing these elements.
- Write a PHP function to slice an array based on an input parameter n and return the resulting subarray.
- Write a PHP program to validate the value of n and then use array_slice() to form a new array from the first n strings.
- Write a PHP script to manually loop through an array and extract the first n strings into a new array.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to count the number of strings with given length in given array of strings.
Next: Write a PHP program to check a positive integer and return true if it contains a number 2.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.