PHP Exercises: Check the largest number among three given integers
18. Largest of Three Integers
Write a PHP program to check the largest number among three given integers.
Sample Solution:
PHP Code :
<?php
// Define a function that takes three parameters and returns the maximum value among them
function test($x, $y, $z)
{
// Find the maximum value using the max function, first comparing $x and $y, and then comparing the result with $z
$max = max($x, max($y, $z));
return $max;
}
// Test the function with different sets of values
echo test(1, 2, 3)."\n";
echo test(1, 3, 2)."\n";
echo test(1, 1, 1)."\n";
echo test(1, 2, 2)."\n";
?>
Explanation:
- Function Definition:
- The test function takes three parameters: $x, $y, and $z.
- Finding the Maximum Value:
- It calculates the maximum value among the three parameters by using the max function.
- First, it finds the maximum between $y and $z using max($y, $z).
- Then, it compares the result with $x to get the overall maximum value, storing it in $max.
- Return Statement:
- The function returns the value of $max.
- Function Calls and Output:
- First Call: test(1, 2, 3) returns 3 as the highest value.
- Second Call: test(1, 3, 2) returns 3 as the highest value.
- Third Call: test(1, 1, 1) returns 1 since all values are equal.
- Fourth Call: test(1, 2, 2) returns 2 as the highest value.
Output:
3 3 1 2
Visual Presentation:
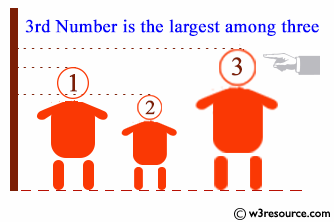
Flowchart:
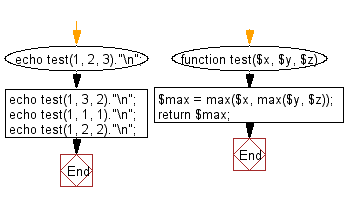
For more Practice: Solve these Related Problems:
- Write a PHP script to compare three integers and return the largest value using nested ternary operators.
- Write a PHP function to determine the maximum among three numbers without using built-in max() function.
- Write a PHP program to compare three user inputs and output the greatest integer with error checking.
- Write a PHP script to apply an iterative method to find the maximum value from three given numbers.
Go to:
PREV : Remove "yt" from Index 1.
NEXT : Nearest to 100 Check.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.