PHP Exercises: Check which number nearest to the value 100 among two given integers
19. Nearest to 100 Check
Write a PHP program to check which number nearest to the value 100 among two given integers. Return 0 if the two numbers are equal.
Sample Solution:
PHP Code :
<?php
// Define a function that takes two parameters and returns the one closer to the value 100
function test($x, $y)
{
// Define the target value
$n = 100;
// Calculate the absolute difference between $x and $n
$val = abs($x - $n);
// Calculate the absolute difference between $y and $n
$val2 = abs($y - $n);
// Compare the absolute differences and return the value closer to $n
return $val == $val2 ? 0 : ($val < $val2 ? $x : $y);
}
// Test the function with different sets of values
echo test(78, 95)."\n";
echo test(95, 95)."\n";
echo test(99, 70)."\n";
?>
Explanation:
- Function Definition:
- The test function takes two parameters, $x and $y.
- Target Value:
- A variable $n is defined and set to 100, representing the target value to compare against.
- Absolute Differences:
- The absolute difference between $x and $n is calculated and stored in $val.
- The absolute difference between $y and $n is calculated and stored in $val2.
- Comparison and Return:
- If $val (difference of $x from 100) equals $val2 (difference of $y from 100), the function returns 0 (indicating both are equally close).
- If $val is smaller than $val2, the function returns $x (as it’s closer to 100).
- Otherwise, it returns $y (indicating $y is closer to 100).
Output:
95 0 99
Pictorial Presentation:
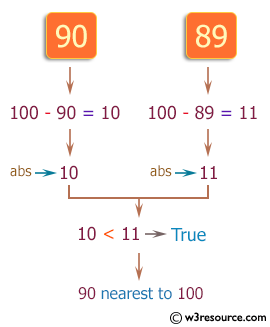

Flowchart:
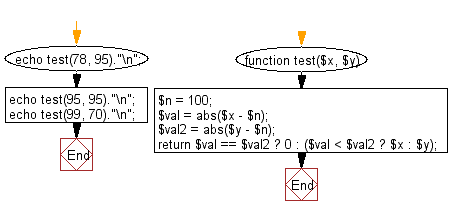
For more Practice: Solve these Related Problems:
- Write a PHP function to determine which of two integers is closer to 100, returning 0 if they are equidistant.
- Write a PHP script to compute the absolute difference from 100 for two numbers and compare the results for proximity.
- Write a PHP program to calculate and return the integer nearest to 100, handling tie cases explicitly.
- Write a PHP script to implement a comparison mechanism that outputs the number closer to 100 using conditional operators.
Go to:
PREV : Largest of Three Integers.
NEXT : Check Integers in Range 40..50 or 50..60.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.