PHP Exercises: Create a string like "aababcabcd" from a given string "abcd"
30. Build Progressive String Pattern
Write a PHP program to create a string like "aababcabcd" from a given string "abcd".
Sample Solution:
PHP Code :
<?php
// Define a function that generates a string by concatenating substrings from the input string
function test($s)
{
// Initialize an empty string to store the result
$result = "";
// Iterate through the string
for ($i = 0; $i < strlen($s); $i++) {
// Append substrings from the beginning up to the current index
$result .= substr($s, 0, $i + 1);
}
// Return the final result
return $result;
}
// Test the function with different input strings
echo test("abcd")."\n";
echo test("abc")."\n";
echo test("a")."\n";
?>
Explanation:
- Function Definition:
- The test function takes a single parameter, $s, which is a string. It builds and returns a new string by concatenating progressively longer substrings from $s.
- Initialize Result Variable:
- An empty string $result is initialized to store the concatenated substrings.
- Iterate Through String:
- A for loop iterates through each character of the string $s, from index 0 to strlen($s) - 1.
- Append Substrings:
- For each index $i, a substring from the beginning of $s up to the character at position $i is appended to $result.
- substr($s, 0, $i + 1) extracts this substring.
- Return Final Result:
- After the loop, $result contains the cumulative substrings and is returned.
Output:
aababcabcd aababc a
Flowchart:
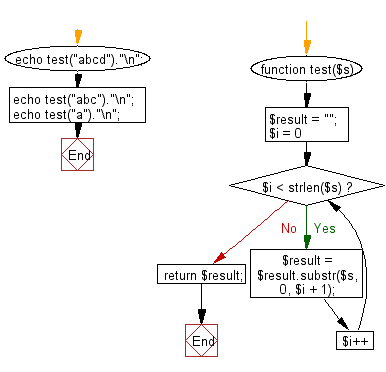
For more Practice: Solve these Related Problems:
- Write a PHP script to generate a pattern string that builds progressively by appending substrings of increasing length.
- Write a PHP function to create a new string that concatenates the first n characters for each n from 1 to the length of the string.
- Write a PHP program to form a pattern where each segment of the original string is progressively added to the result.
- Write a PHP script to use loops to produce a cumulative pattern string from an input word, testing with various lengths.
Go to:
PREV : Every Other Character String.
NEXT : Count Substring Occurrences Excluding End.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.