PHP Exercises: Count a substring of length 2 appears in a given string and also as the last 2 characters of the string
31. Count Substring Occurrences Excluding End
Write a PHP program to count a substring of length 2 appears in a given string and also as the last 2 characters of the string. Do not count the end substring.
Sample Solution:
PHP Code :
<?php
// Define a function that counts the occurrences of the last two characters in substrings of the input string
function test($s)
{
// Extract the last two characters of the input string
$last_two_char = substr($s, strlen($s)-2, 2);
// Initialize a counter variable
$ctr = 0;
// Iterate through the string (excluding the last two characters)
for ($i = 0; $i < strlen($s)-2; $i++) {
// Check if the current substring matches the last two characters
if (substr($s, $i, 2) == $last_two_char) {
// Increment the counter
$ctr = $ctr + 1;
}
}
// Return the final count
return $ctr;
}
// Test the function with different input strings
echo test("abcdsab")."\n";
echo test("abcdabab")."\n";
echo test("abcabdabab")."\n";
echo test("abcabd")."\n";
?>
Explanation:
- Function Definition:
- The test function takes a string $s and counts occurrences of its last two characters within the rest of the string.
- Extract Last Two Characters:
- The last two characters of $s are extracted and stored in $last_two_char using substr($s, strlen($s) - 2, 2).
- Initialize Counter:
- A counter variable $ctr is initialized to zero to keep track of occurrences.
- Iterate Through String:
- A for loop iterates over $s from the beginning to the second-last character (i.e., strlen($s) - 2).
- For each position $i, it checks if the substring from $i of length 2 matches $last_two_char.
- Increment Counter:
- If a match is found, $ctr is incremented by one.
- Return Final Count:
- After the loop, the function returns $ctr, which is the count of occurrences.
Output:
1 2 3 0
Visual Presentation:
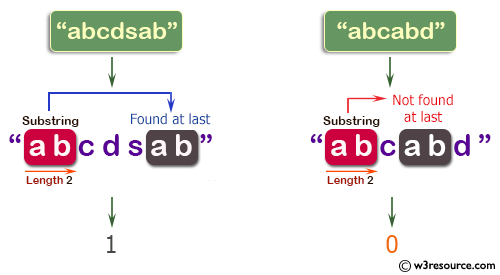
Flowchart:
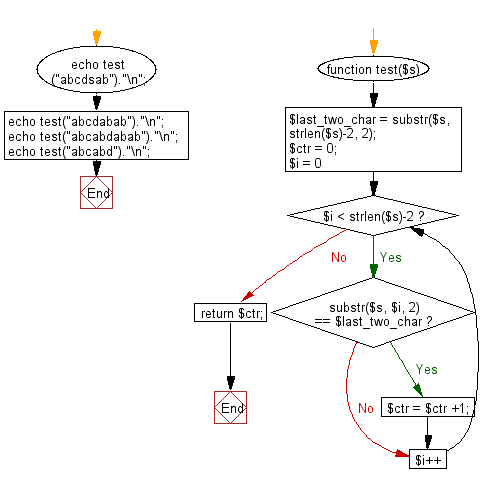
For more Practice: Solve these Related Problems:
- Write a PHP script to count how many times the last two characters appear in a string, excluding their occurrence at the end.
- Write a PHP function to extract the last two characters and scan the rest of the string for additional occurrences.
- Write a PHP program to tally non-overlapping occurrences of a target substring that matches the final two characters, ignoring the last instance.
- Write a PHP script to implement substring counting using string functions and manually exclude the final occurrence from the count.
Go to:
PREV : Build Progressive String Pattern.
NEXT : Specified Number in Array Check.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.