PHP Exercises: Check a specified number is present in a given array of integers
32. Specified Number in Array Check
Write a PHP program to check a specified number is present in a given array of integers.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if a given number is present in an array of numbers
function test($nums, $n)
{
// Use in_array function to check if the number is present in the array
if (in_array($n, $nums)) {
return true;
}
// Return false if the number is not found in the array
return false;
}
// Test the function with different arrays and numbers
var_dump(test(array(1,2,9,3), 3));
var_dump(test(array(1,2,2,3), 2));
var_dump(test(array(1,2,2,3), 9));
?>
Explanation:
- Function Definition:
- The test function takes two parameters: an array of numbers $nums and a number $n to check for in the array.
- Check for Number in Array:
- The in_array() function is used to check if $n is present in $nums.
- If $n is found, the function returns true.
- Return False if Not Found:
- If $n is not in $nums, the function returns false.
Output:
bool(true) bool(true) bool(false)
Visual Presentation:
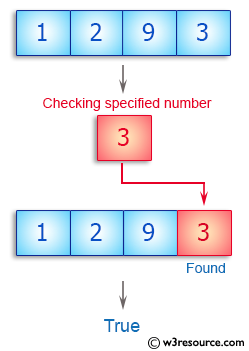
Flowchart:
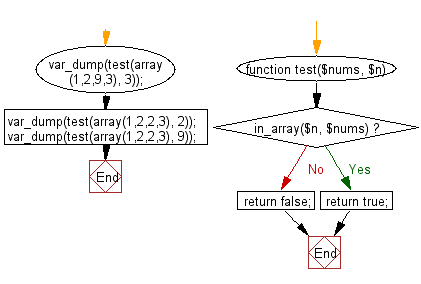
For more Practice: Solve these Related Problems:
- Write a PHP script to check whether a specified number exists within an array of integers using a loop.
- Write a PHP function to determine the presence of a target integer in an array without using in_array().
- Write a PHP program to iterate over an array and return a boolean based on the existence of a given value.
- Write a PHP script to simulate a search for a number in an array with manual iteration and conditional comparisons.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to count a substring of length 2 appears in a given string and also as the last 2 characters of the string. Do not count the end substring.
Next: Write a PHP program to check if one of the first 4 elements in an array of integers is equal to a given element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.