PHP Exercises: Check three given integers and return true if one of them is 20 or more less than one of the others
50. Check if One Integer is 20 or More Less Than Another
Write a PHP program to check three given integers and return true if one of them is 20 or more less than one of the others.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if the absolute difference between two numbers is greater than or equal to 20
function test($x, $y, $z)
{
// Check if the absolute difference between $x and $y is greater than or equal to 20,
// or if the absolute difference between $x and $z is greater than or equal to 20,
// or if the absolute difference between $y and $z is greater than or equal to 20
return abs($x - $y) >= 20 || abs($x - $z) >= 20 || abs($y - $z) >= 20;
}
// Test the function with different sets of numbers
var_dump(test(11, 21, 31))."\n";
var_dump(test(11, 22, 31))."\n";
var_dump(test(10, 20, 15))."\n";
?>
Explanation:
- Function Definition:
- The function test checks if the absolute difference between any two of the three numbers $x, $y, or $z is greater than or equal to 20.
- Condition Checked:
- The function calculates the absolute difference between:
- $x and $y
- $x and $z
- $y and $z
- It then checks if any of these differences are >= 20.
Output:
bool(true) bool(true) bool(false)
Visual Presentation:
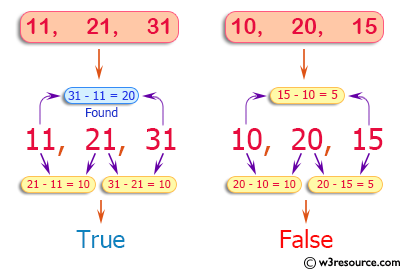
Flowchart:
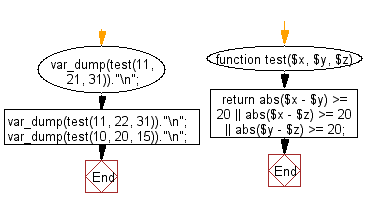
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check if two or more non-negative given integers have the same rightmost digit.
Next: Write a PHP program to find the larger from two given integers. However if the two integers have the same remainder when divided by 7, then the return the smaller integer. If the two integers are the same, return 0.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.