PHP Exercises: Find the larger from two given integers
51. Larger with Remainder Rule
Write a PHP program to find the larger from two given integers. However if the two integers have the same remainder when divided by 7, then the return the smaller integer. If the two integers are the same, return 0.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that compares two numbers and returns the result
function test($x, $y)
{
// Check if $x is equal to $y
if ($x == $y)
{
// Return 0 if $x is equal to $y
return 0;
}
// Check if $x is not equal to $y and satisfies another condition
else if (($x % 7 == $y % 7 && $x < $y) || $x > $y)
{
// Return $x if the condition is met
return $x;
}
// If none of the above conditions are met
else
{
// Return $y
return $y;
}
}
// Test the 'test' function with different input values and display the results
echo test(11, 21)."\n";
echo test(11, 20)."\n";
echo test(10, 10)."\n";
?>
Explanation:
- Function Definition:
- The function test compares two numbers, $x and $y, and returns a result based on specific conditions.
- Conditions and Logic:
- Condition 1: If $x is equal to $y, it returns 0.
- Condition 2: If $x is not equal to $y, it checks:
- If $x and $y have the same remainder when divided by 7 ($x % 7 == $y % 7) and $x is less than $y, or if $x is greater than $y, then it returns $x.
- Condition 3: If none of the above conditions are met, it returns $y.
Output:
21 20 0
Flowchart:
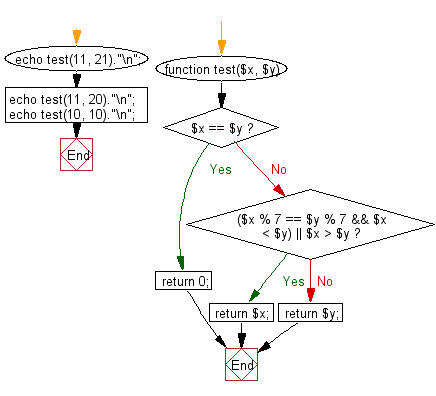
For more Practice: Solve these Related Problems:
- Write a PHP script to compare two integers and return the larger value unless both yield the same remainder when divided by 7, then output the smaller.
- Write a PHP function that accepts two numbers, compares their remainders modulo 7, and conditionally returns the larger or smaller based on the remainder equality.
- Write a PHP program that evaluates two integers and if they have equal modulo 7 remainders, outputs the lesser value; otherwise, outputs the greater one.
- Write a PHP script to design a function which returns 0 if both integers are equal, the smaller one if remainders match, and the larger one otherwise.
Go to:
PREV : Check if One Integer is 20 or More Less Than Another.
NEXT : Shared Digit in Two Integers.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.