PHP Exercises: Compute the sum of the three given integers. However, if any of the values is in the range 10..20 inclusive then that value counts as 0, except 13 and 17
56. Sum with Range Nullification Except 13/17
Write a PHP program to compute the sum of the three given integers. However, if any of the values is in the range 10..20 inclusive then that value counts as 0, except 13 and 17.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes three parameters and returns the sum of fixed numbers
function test($x, $y, $z)
{
// Call the 'fix_num' function on each input parameter and return the sum of fixed numbers
return fix_num($x) + fix_num($y) + fix_num($z);
}
// Define a function named 'fix_num' that takes a number as input and returns a fixed number based on conditions
function fix_num($n)
{
// Check if $n is between 10 and 13 or between 17 and 21 (exclusive)
// If true, return 0; otherwise, return the original number
return (($n < 13 && $n > 9) || ($n > 17 && $n < 21)) ? 0 : $n;
}
// Test the 'test' function with different input values and display the results
echo (test(4, 5, 7))."\n";
echo (test(7, 4, 12))."\n";
echo (test(10, 13, 12))."\n";
echo (test(17, 12, 18))."\n";
?>
Explanation:
- Main Function:
- The test function takes three parameters: $x, $y, and $z.
- It calls the fix_num function on each parameter and returns the sum of the resulting values.
- Helper Function (fix_num):
- The fix_num function checks each parameter ($n) to determine if it should be set to 0 based on specific ranges:
- If $n is between 10 and 13 (exclusive) or between 17 and 21 (exclusive), it returns 0.
- Otherwise, it returns the original value of $n.
Output:
16 11 13 17
Flowchart:
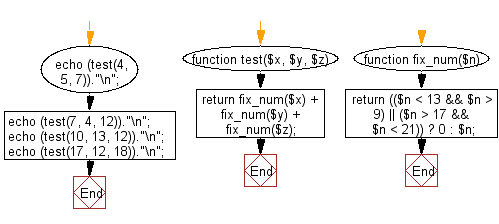
For more Practice: Solve these Related Problems:
- Write a PHP script to sum three integers while setting any value in the range 10 to 20 to 0, except if it is 13 or 17.
- Write a PHP function that evaluates three numbers and replaces those within a specific range with zero, with exceptions for designated values.
- Write a PHP program to conditionally zero out numbers in a triplet if they fall between 10 and 20 (except for 13 and 17) before summing.
- Write a PHP script to incorporate range filtering on three inputs and then compute their sum after exempting the special cases.
Go to:
PREV : Sum Excluding 13 and Right Side.
NEXT : Nearest to 13 Without Overrun.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.