PHP Exercises: Check two given integers and return the value whichever value is nearest to 13 without going over
PHP Basic Algorithm: Exercise-57 with Solution
Write a PHP program to check two given integers and return the value whichever value is nearest to 13 without going over. Return 0 if both numbers go over.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes two parameters and returns a value based on conditions
function test($x, $y)
{
// Check if both $x and $y are greater than 13; if true, return 0
if ($x > 13 && $y > 13) return 0;
// Check if $x is less than or equal to 13 and $y is greater than 13; if true, return $x
if ($x <= 13 && $y > 13) return $x;
// Check if $y is less than or equal to 13 and $x is greater than 13; if true, return $y
if ($y <= 13 && $x > 13) return $y;
// If none of the above conditions are met, return the greater of $x and $y
return $x > $y ? $x : $y;
}
// Test the 'test' function with different input values and display the results
echo (test(4, 5))."\n";
echo (test(7, 12))."\n";
echo (test(10, 13))."\n";
echo (test(17, 33))."\n";
?>
Explanation:
- Function Purpose:
- The test function takes two parameters, $x and $y, and returns a value based on several conditions involving the numbers' relationship to 13.
- Conditions:
- If both $x and $y are greater than 13, it returns 0.
- If $x is less than or equal to 13 and $y is greater than 13, it returns $x.
- If $y is less than or equal to 13 and $x is greater than 13, it returns $y.
- If none of the above conditions are met, it returns the greater of $x and $y.
Output:
5 12 13 0
Flowchart:
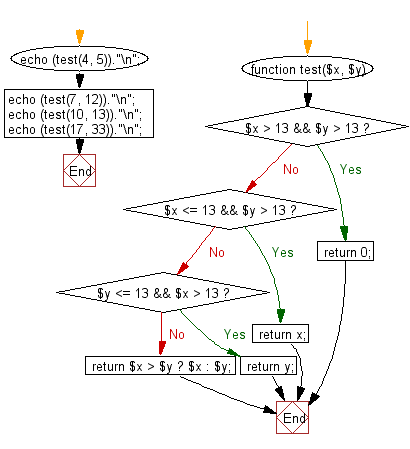
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compute the sum of the three given integers. However, if any of the values is in the range 10..20 inclusive then that value counts as 0, except 13 and 17.
Next: Write a PHP program to check three given integers (small, medium and large) and return true if the difference between small and medium and the difference between medium and large is same.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-57.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics