PHP Exercises: Check three given integers and return true if the difference between small and medium and the difference between medium and large is same
58. Equal Differences in Three Integers
Write a PHP program to check three given integers (small, medium and large) and return true if the difference between small and medium and the difference between medium and large is same.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes three parameters and returns a boolean value based on conditions
function test($x, $y, $z)
{
// Check if the sequence of numbers is in ascending order and the differences are equal
if ($x > $y && $x > $z && $y > $z) return $x - $y == $y - $z;
// Check if the sequence of numbers is in ascending order and the differences are equal
if ($x > $y && $x > $z && $z > $y) return $x - $z == $z - $y;
// Check if the sequence of numbers is in ascending order and the differences are equal
if ($y > $x && $y > $z && $x > $z) return $y - $x == $x - $z;
// Check if the sequence of numbers is in ascending order and the differences are equal
if ($y > $x && $y > $z && $z > $x) return $y - $z == $z - $x;
// Check if the sequence of numbers is in ascending order and the differences are equal
if ($z > $x && $z > $y && $x > $y) return $z - $x == $x - $y;
// If none of the above conditions are met, check if the sequence of numbers is in ascending order and the differences are equal
return $z - $y == $y - $x;
}
// Test the 'test' function with different input values and display the results
var_dump(test(4, 5, 6));
var_dump(test(7, 12, 13));
var_dump(test(-1, 0, 1));
?>
Explanation:
- Function Purpose:
- The test function takes three numbers as parameters ($x, $y, $z) and checks if they form an ordered sequence (in ascending or descending order) where the difference between consecutive numbers is the same.
- Conditions:
- The function checks different possible sequences and returns true if the difference between consecutive numbers is equal:
- Condition 1: $x > $y > $z, and the difference between $x - $y equals $y - $z.
- Condition 2: $x > $z > $y, and the difference between $x - $z equals $z - $y.
- Condition 3: $y > $x > $z, and the difference between $y - $x equals $x - $z.
- Condition 4: $y > $z > $x, and the difference between $y - $z equals $z - $x.
- Condition 5: $z > $x > $y, and the difference between $z - $x equals $x - $y.
- Condition 6: If none of the above match, it checks if $z > $y > $x with the difference between $z - $y equaling $y - $x.
Output:
bool(true) bool(false) bool(true)
Flowchart:
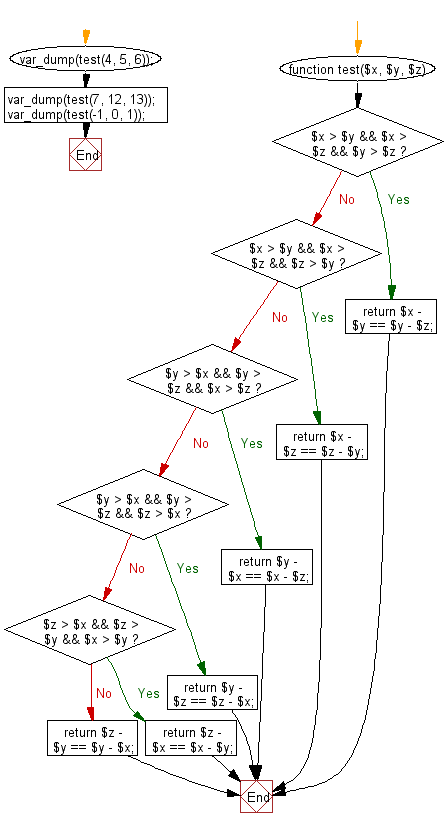
For more Practice: Solve these Related Problems:
- Write a PHP script to check if the difference between the smallest and middle integers equals the difference between the middle and largest.
- Write a PHP function to determine if three numbers form an arithmetic sequence by comparing successive differences.
- Write a PHP program to compute the differences between a sorted triplet and verify if they are identical.
- Write a PHP script to use subtraction on three inputs and return true if the gap between the first two equals that of the last two.
Go to:
PREV : Nearest to 13 Without Overrun.
NEXT : String Pattern: s1s2s2s.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.