PHP Exercises: Create a new string using 3 copies of the first 2 characters of a given string
82. Three Copies of First Two Characters
Write a PHP program to create a new string using 3 copies of the first 2 characters of a given string. If the length of the given string is less than 2 use the whole string.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that repeats the first two characters of a string
function test($s1)
{
// Initialize a variable to store the repeated characters
$extra_Front = "";
// Check if the length of $s1 is less than 2
if (strlen($s1) < 2)
{
// If so, concatenate $s1 with itself and return the result
return $s1 . $s1 . $s1;
}
// If the length of $s1 is 2 or more
else
{
// Extract the first two characters of $s1
$extra_Front = substr($s1, 0, 2);
// Concatenate the extracted characters with themselves and return the result
return $extra_Front . $extra_Front . $extra_Front;
}
}
// Test the 'test' function with different strings, then display the results
echo test("abc") . "\n";
echo test("Python") . "\n";
echo test("J") . "\n";
?>
Explanation:
- Function Definition:
- The function test is defined with one parameter:
- $s1: the input string.
- Variable Initialization:
- A variable $extra_Front is initialized as an empty string to store the first two characters if needed.
- Length Check:
- The function checks the length of $s1 to determine how to process it.
- Condition Handling:
- Case 1: If the length of $s1 is less than 2:
- The function returns the string concatenated with itself three times:
- return $s1 . $s1 . $s1;
- This means that if the input is a single character or empty, it will repeat that character or string three times.
- Case 2: If the length of $s1 is 2 or more:
- The function extracts the first two characters of $s1 using:
- $extra_Front = substr($s1, 0, 2);
- The function then returns these two characters concatenated with themselves three times:
- return $extra_Front . $extra_Front . $extra_Front;
Output:
ababab PyPyPy JJJ
Flowchart:
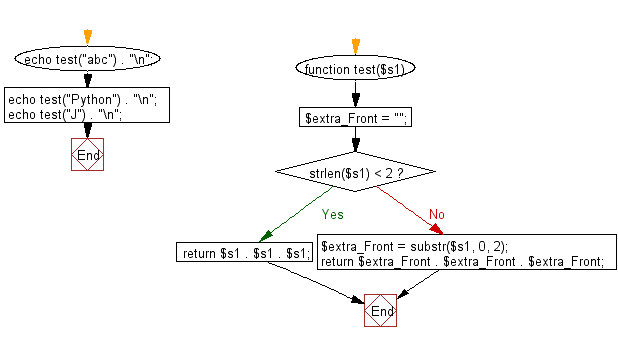
For more Practice: Solve these Related Problems:
- Write a PHP script to generate a new string by repeating the first two characters of an input three times, using the whole string if its length is less than 2.
- Write a PHP function to check the length of a string and then output three copies of its first two characters or the entire string if shorter.
- Write a PHP program to create a repeated pattern from the first two letters of a string and handle edge cases for short inputs.
- Write a PHP script to conditionally replicate the initial segment of a string three times and display the result.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to concat two given strings. If the given strings have different length remove the characters from the longer string.
Next: Write a PHP program to create a new string from a given string. If the two characters of the given string from its beginning and end are same return the given string without the first two characters otherwise return the original string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.